Non Weighted Code Example
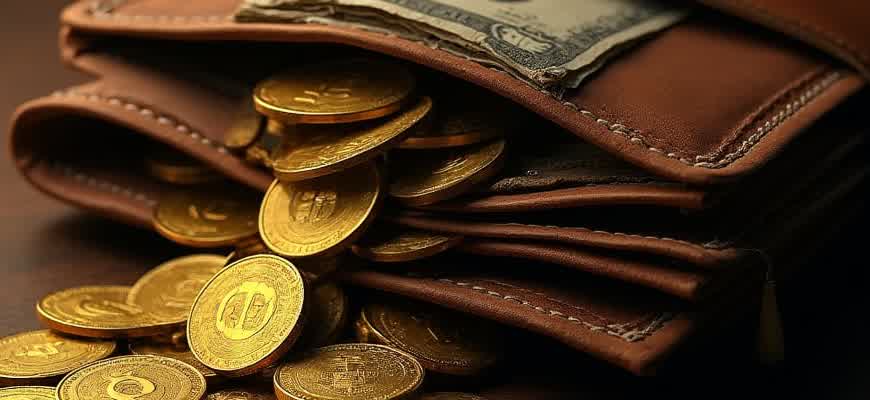
A non-weighted code refers to a coding scheme where each symbol in the code has the same length, without any variation in the number of bits used to represent different symbols. This type of coding is used in scenarios where equal representation is essential, and no symbol is assigned more or fewer bits than others.
In this example, we will demonstrate how a simple non-weighted code can be implemented using basic constructs in programming. The following list shows how different characters are encoded using this scheme:
- A - 000
- B - 001
- C - 010
- D - 011
- E - 100
The code structure is simple: each symbol is represented by exactly three bits, regardless of its frequency of occurrence. This makes the code non-weighted. Below is a basic implementation in a tabular format:
Symbol | Binary Code |
---|---|
A | 000 |
B | 001 |
C | 010 |
D | 011 |
E | 100 |
Note: In this type of coding, all symbols are treated equally, with no regard to their occurrence frequencies, making the system simple but potentially inefficient in terms of compression.
Integrating Non-Weighted Code into Your Workflow
Integrating non-weighted code into your existing development pipeline can enhance efficiency without introducing unnecessary complexity. Non-weighted code generally focuses on simplicity and direct implementation, which can be easily integrated into pre-existing frameworks or projects. The key is understanding how it fits into the logic of the workflow without disrupting the current system or requiring major adjustments. By using a minimalistic approach, developers can keep the flow of work consistent while benefiting from faster execution and easier maintenance.
To ensure smooth integration, follow a few key steps. First, thoroughly evaluate your existing codebase and identify areas where lightweight solutions can be applied. Then, follow a structured approach to introduce non-weighted code in such a way that it complements, rather than competes with, your current implementation. Below are steps for successful integration.
Steps to Seamlessly Integrate Non-Weighted Code
- Evaluate Current Architecture: Understand the existing system’s logic and identify modules where non-weighted code can be introduced without disrupting performance.
- Prototype Small Segments: Start by creating small, isolated units of non-weighted code. Test them separately before integrating them into the main workflow.
- Ensure Compatibility: Review dependencies and ensure that the non-weighted code aligns with existing frameworks and libraries.
- Maintain Scalability: While the code is lightweight, ensure that it is scalable for future growth and that it doesn’t limit your ability to expand the system.
- Test Rigorously: Conduct thorough testing to ensure that the new code does not introduce bugs or unexpected behavior.
Example Workflow with Non-Weighted Code Integration
Step | Action | Expected Outcome |
---|---|---|
1 | Prototype non-weighted code | Fast implementation without overhead |
2 | Test in isolation | Verify that the solution works independently |
3 | Integrate into existing flow | Ensure no conflicts with the current system |
Note: Non-weighted code can often be more fragile when dealing with more complex workflows, so it's crucial to regularly monitor its performance after integration.
Common Errors When Working with Unweighted Code and How to Prevent Them
Unweighted code is commonly used in algorithms where the cost or importance of the edges, or operations, are not considered. While it offers a simplified approach, many developers fall into traps that lead to inefficient or incorrect results. Recognizing these mistakes is crucial to ensure optimal performance and accuracy.
One of the most frequent errors is assuming that the absence of weights means equal treatment of all paths. This misinterpretation can lead to poor design choices, particularly in graph traversal and search algorithms. Avoiding this mistake requires a clear understanding of the problem and the constraints that define unweighted approaches.
1. Ignoring Edge Connectivity
When dealing with unweighted graphs or networks, developers may overlook the importance of edge connectivity. Treating all edges equally can result in inefficient traversal or incomplete solutions.
Important: Always ensure that the edges are well-connected and appropriately explored in algorithms like BFS or DFS.
2. Inaccurate Assumptions About Path Length
Another common issue arises when developers assume all paths are the same length in unweighted scenarios. This leads to faulty calculations, especially in shortest path problems.
- Example: Assuming that a path with more nodes has the same weight as a direct path.
- Solution: Use breadth-first search (BFS) when solving for shortest paths in unweighted graphs to avoid such mistakes.
3. Overlooking Edge Direction in Directed Graphs
In directed graphs, developers might forget to account for edge direction, treating all edges as bidirectional. This can result in incorrect results or infinite loops.
Graph Type | Issue | Solution |
---|---|---|
Undirected | Assuming bidirectionality where it's not present | Ensure correct edge traversal and directionality handling. |
Directed | Ignoring the direction of edges | Respect edge directionality during traversal and path finding. |
4. Not Optimizing Algorithm Efficiency
Using unweighted code without considering algorithmic efficiency can lead to unnecessary computational overhead, especially in large graphs.
Tip: Use BFS for finding the shortest path in unweighted graphs rather than more complex algorithms like Dijkstra.
Enhancing Performance with Unweighted Logic in Code
When working with unweighted logic, the goal is to streamline the execution of algorithms without introducing complex data structures or calculations that would otherwise impact performance. In this approach, optimizing the performance focuses on eliminating unnecessary computations and simplifying decision-making processes. The primary challenge here lies in minimizing the use of resources while maintaining the integrity and correctness of the logic.
One key aspect of improving performance involves reducing the complexity of conditions and calculations. In unweighted logic, avoiding the use of excessive condition checks or loops can significantly enhance the speed of execution. Below are some strategies to achieve this optimization:
Techniques for Optimizing Code
- Minimize Condition Checks: Avoid redundant evaluations of the same conditions within loops or functions.
- Use Efficient Data Structures: Choose the simplest data structures that still meet the algorithm’s requirements, such as arrays instead of linked lists.
- Short-circuit Evaluation: Leverage logical operators like AND/OR to stop evaluations as soon as a decision can be made.
Example Code:
Example of an optimized loop with unweighted logic:
for (int i = 0; i < n; i++) { if (arr[i] == target) { return i; } }
As shown in the example above, the loop stops as soon as the target is found, avoiding unnecessary iterations. This is a simple yet effective technique for improving performance.
Comparing Different Approaches
Method | Performance Impact | Use Case |
---|---|---|
Redundant Checks | Negative | Unnecessary condition checks inside loops |
Short-Circuiting | Positive | Early termination of checks in boolean expressions |
Efficient Data Structures | Positive | Using minimal, purpose-driven data structures |
Testing Unweighted Code: Best Practices for Ensuring Accuracy
When working with unweighted code, ensuring the reliability of your test cases is crucial. Unlike weighted algorithms, where elements have assigned values affecting the output, unweighted code can introduce complexities that require specific attention. Testing for accuracy in such cases can sometimes be challenging due to the absence of explicit value assignments, making it necessary to implement specific strategies for validation.
To ensure your tests are effective and provide accurate results, a structured approach is essential. The following practices will help ensure your unweighted code performs as expected under various scenarios. It's important to include comprehensive checks, edge case handling, and consistency across different inputs.
Best Practices
- Define Clear Expectations: Understand the logic and expected output for each input scenario. This will provide a baseline for testing.
- Use Unit Tests: Break down your code into smaller components and test each part individually to identify potential errors.
- Check for Edge Cases: Always test your code with boundary values and other extreme inputs to verify its robustness.
Testing Approach
- Test for Correctness: Ensure that your code returns the correct results for all possible inputs, including empty sets and large datasets.
- Test Performance: Even though unweighted code may not involve complex calculations, always check its performance under varying loads.
- Ensure Code Coverage: Make sure your tests cover all branches and conditions in the code, especially any conditional logic.
Testing unweighted code requires a strong focus on edge cases and input validation to ensure accuracy. Small mistakes can lead to unexpected results, making comprehensive testing essential.
Sample Test Matrix
Test Case | Expected Result | Notes |
---|---|---|
Empty Input | No Output | Ensure that the function handles an empty input gracefully. |
Large Input Size | Correct Output | Test the performance when dealing with large data. |
Negative Values | Handle Correctly | Check how the code handles negative or unusual values. |
Adapting Non-Weighted Algorithms for Complex Data Structures
When working with complex data structures, adapting non-weighted algorithms often requires specific modifications to account for additional dimensions of data. Non-weighted approaches, by design, treat all elements or nodes equally, which is typically suitable for simple tasks like searching or sorting. However, more intricate structures, such as trees, graphs, or multi-dimensional arrays, introduce complexities that challenge these methods. To address these, one must extend the basic logic of non-weighted algorithms to handle various levels of hierarchy and connections.
One common method to adapt non-weighted code for complex data types involves representing relationships or connections explicitly within the structure. For instance, when dealing with a graph, each edge may need to be processed sequentially, even if the algorithm remains non-weighted. In this case, you would modify the traversal logic, such as Depth-First Search (DFS) or Breadth-First Search (BFS), to consider the structure's multiple dimensions while maintaining the uniform treatment of data points.
Techniques for Modifying Non-Weighted Algorithms
- Representing Data Connections: Ensure that relationships between nodes or elements are clearly defined, even if the connection strength is not a factor.
- Traversal Adjustments: Modify search algorithms like BFS and DFS to account for complex data hierarchies, without introducing weights.
- Handling Multiple Data Types: When structures contain various data types (e.g., a combination of arrays, objects, and lists), adapt the algorithm to handle these without prioritizing one over the other.
"The key challenge when adapting non-weighted algorithms is to maintain simplicity without losing the capacity to navigate through intricate data structures."
- Modify traversal methods to support nested data structures.
- Ensure that each node is processed uniformly despite its position in the structure.
- Test performance to ensure that the adapted algorithm does not introduce unnecessary overhead.
Example Adjustment for Tree Structure
Step | Action |
---|---|
1 | Initialize traversal, ensuring each node is visited without bias to its depth. |
2 | Adapt BFS or DFS to handle branches and subtrees efficiently. |
3 | Ensure all nodes are processed uniformly, without weighting edge traversal. |
Comparison of Non-Weighted Code and Traditional Weighted Approaches
In software development, various techniques are employed to make decisions about which components to prioritize in a program. One approach is using non-weighted code, where each decision or feature is treated with equal importance, without distinguishing between their relative impact. This method contrasts with traditional weighted approaches, where certain aspects are given more significance based on predefined criteria or data analysis.
The key difference lies in how priorities are assigned to different factors. Non-weighted code simplifies the development process but may lack the precision required for optimizing performance in complex systems. Weighted methods, on the other hand, offer more control over performance and resource allocation but require more detailed input and analysis.
Advantages of Non-Weighted vs. Weighted Approaches
- Non-Weighted Code:
- Simplicity in implementation.
- Faster to develop and easier to maintain.
- Less prone to overfitting due to fewer parameters to tune.
- Weighted Approaches:
- Allows for more granular control of system behavior.
- Better suited for systems where certain factors are more important.
- Can optimize resource usage and performance more effectively.
Key Differences in Performance Optimization
While non-weighted methods are simpler and can be faster to implement, they might lead to suboptimal performance when applied to complex or large-scale systems. In contrast, weighted methods offer the ability to assign varying levels of importance to different variables, potentially leading to more efficient algorithms or processes.
Weighted methods provide precision, but at the cost of increased complexity.
Example of Non-Weighted and Weighted Approaches
Approach | Example | Benefits |
---|---|---|
Non-Weighted | Basic search algorithm without priorities | Simplicity, ease of implementation |
Weighted | Search algorithm prioritizing important variables like relevance | More accurate results, optimization of resources |
Managing Code Maintainability with Non Weighted Methods
Effective management of code maintainability is crucial for long-term software success. Using methods that do not rely on weighted metrics can help simplify the process, focusing on clarity and consistency in the code. This approach is beneficial for teams who prioritize clean, understandable code over complex evaluations. It reduces the need for heavy calculations and allows developers to focus on core functionality, ensuring code remains accessible for future updates and improvements.
Incorporating non-weighted methods can significantly reduce technical debt. By using these methods, teams can streamline their development processes, making it easier to adapt to changes without over-complicating the codebase. Non-weighted techniques ensure that every change is evaluated based on fundamental principles, avoiding the trap of over-engineering or unnecessary optimizations.
Advantages of Non-Weighted Approaches
- Simplicity: Code remains easy to understand and modify, even for new team members.
- Consistency: Non-weighted methods encourage uniformity, reducing the risk of divergent coding practices within the team.
- Flexibility: These methods allow for quick changes without the need to adjust complex weight-based evaluations.
Practical Applications
- Refactoring: Simplifying legacy code without focusing on complex performance metrics.
- Testing: Ensuring the correctness of code without relying heavily on scoring systems or performance benchmarks.
- Documentation: Writing clear and concise documentation that reflects the true functionality without unnecessary details.
Considerations When Using Non-Weighted Techniques
Factor | Impact |
---|---|
Code Complexity | Non-weighted methods may lead to more verbose code in some situations. |
Performance | These methods might not address performance optimization in detail. |
Future Scalability | While non-weighted methods simplify maintenance, they may need adjustments as the project grows. |
"Using non-weighted approaches can foster a more approachable codebase, but careful attention is needed as complexity and requirements grow."
Real-World Applications of Non-Weighted Code in Industry
Non-weighted algorithms and their corresponding code implementations play an important role in a wide variety of industrial applications. In simple terms, non-weighted algorithms are used in scenarios where each data point, or node, in a structure such as a graph or network has equal significance. This approach is common in situations where the goal is to find optimal or approximate solutions based on simple, unweighted parameters, rather than relying on complex weighted metrics. Industries like telecommunications, logistics, and finance have seen significant benefits from utilizing non-weighted algorithms in their operations.
By focusing on simpler models, non-weighted code implementations help reduce computational overhead and complexity, making them suitable for real-time systems, or applications where quick approximations are acceptable. Let's explore a few concrete examples of how these systems are used in industry today.
Key Applications in Different Sectors
- Telecommunications: Non-weighted algorithms are used to analyze network topologies, where each connection between nodes (such as routers or switches) is assumed to be of equal importance. For example, algorithms like breadth-first search (BFS) help find the shortest path between two devices in a network.
- Logistics: In supply chain management, non-weighted code can simplify route optimization. For instance, algorithms can be used to identify the most efficient delivery routes without the need for complex cost analysis, making real-time adjustments based on basic distance or time metrics.
- Finance: Fraud detection systems often use non-weighted approaches to quickly identify patterns or connections between transactions. These methods allow for fast preliminary analysis of large data sets before more in-depth, weighted analyses are applied.
Advantages of Non-Weighted Code
- Speed: Non-weighted algorithms typically run faster due to simpler logic and fewer parameters, making them ideal for applications that require real-time processing.
- Scalability: These methods can be scaled to handle large datasets without requiring extensive computational resources, making them cost-effective for large-scale operations.
- Simplicity: The logic behind non-weighted algorithms is often more straightforward, allowing for easier implementation and fewer chances for errors in code.
Example: Network Pathfinding
Algorithm | Purpose | Application |
---|---|---|
Breadth-First Search | Find the shortest path between nodes | Used in telecommunication networks to optimize routing paths |
Depth-First Search | Explore all nodes in a graph | Used in supply chain analysis to map out warehouse systems |
Non-weighted algorithms provide a simple, efficient way to approach complex problems, especially when the goal is to find fast, approximated solutions in real-time environments.