Website Creation Using Tools in Javascript
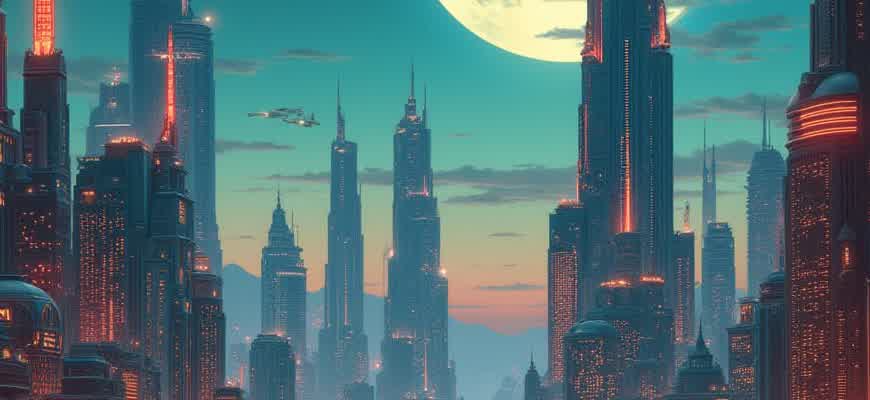
In modern web development, JavaScript plays a crucial role in creating dynamic and interactive websites. There are various tools available that simplify the process, making it easier to build functional and visually appealing websites. These tools enable developers to enhance website performance, manage complex tasks, and streamline the development workflow.
Below are some of the essential tools developers use to create websites with JavaScript:
- Frameworks: Libraries like React, Angular, and Vue.js offer structured approaches to building web applications. These frameworks allow for faster development, with pre-built components and tools for state management.
- Build Tools: Tools like Webpack, Gulp, and Parcel help in bundling JavaScript code, optimizing images, and automating repetitive tasks like minification.
- Code Editors: Modern code editors such as Visual Studio Code or Sublime Text provide features like syntax highlighting, auto-completion, and debugging capabilities, which make coding more efficient.
To better understand the significance of these tools, let’s consider some key aspects of a typical JavaScript-based development setup:
Tool | Purpose | Example |
---|---|---|
React | UI framework for building component-based applications | Single-page applications (SPAs), dynamic websites |
Webpack | Module bundler for optimizing JavaScript code and assets | Optimizing file size for production environments |
Gulp | Task runner for automating build processes | Automating CSS preprocessing, minification |
Utilizing these tools can significantly improve the efficiency and scalability of your web projects.
Choosing the Right JavaScript Framework for Your Website
When planning the development of a website, selecting the appropriate JavaScript framework is a critical step. With numerous frameworks available, each offering its own set of features and capabilities, the decision can be overwhelming. Understanding the core requirements of your website, such as performance, scalability, and ease of use, will guide you toward the right choice. Frameworks like React, Angular, and Vue.js are among the most popular options, but the best choice depends on your project's specific needs.
Some factors to consider include the complexity of the website, the expected growth in traffic, and the development team's expertise. Additionally, understanding the trade-offs of each framework, such as the learning curve or community support, can help you make a well-informed decision. Below is a comparison of some of the most widely-used frameworks in terms of their features and characteristics.
Framework Comparison
Framework | Strengths | Weaknesses |
---|---|---|
React |
|
|
Angular |
|
|
Vue.js |
|
|
Important: It's essential to evaluate not just the features but also the support and maintenance of the framework. Choose one with an active community and regular updates to ensure long-term viability for your project.
In conclusion, selecting the right JavaScript framework requires understanding both the project requirements and the strengths of the framework. For simple, scalable applications, React or Vue.js may be a better fit, while more complex applications with comprehensive features might benefit from Angular’s full stack capabilities.
Enhancing User Interaction with JavaScript Libraries
JavaScript libraries play a key role in simplifying the process of adding dynamic and interactive elements to websites. By leveraging popular libraries such as jQuery, React, or Vue.js, developers can create responsive components with minimal effort. These libraries provide predefined functions and components that reduce the need for writing complex JavaScript code from scratch, enabling faster and more efficient web development.
Interactive elements like buttons, sliders, and forms can be easily customized and made dynamic using JavaScript. Libraries like jQuery UI, for example, offer ready-to-use widgets for building sophisticated interfaces. This allows developers to focus more on the logic and design rather than the implementation of basic functionality.
Creating Interactive Features with jQuery
With jQuery, developers can effortlessly add animations, event handling, and other interactive features. One of the main advantages is its ability to manipulate the DOM and handle events with minimal code. This allows for enhanced user interaction such as hover effects, dropdown menus, and interactive sliders.
Tip: jQuery's simplicity is especially beneficial for smaller projects or when rapid prototyping is needed.
Building Interactive Forms with React
React, another widely-used JavaScript library, allows developers to create highly interactive and reusable components. By managing the state within components, React makes it easy to build forms with dynamic validation, real-time input feedback, and complex user interfaces that update without needing to refresh the entire page.
- State management: React allows for the seamless tracking of user inputs.
- Reusability: Components in React can be reused across different parts of the application.
- Performance: React updates only the components that need to change, improving the website's speed and efficiency.
Example: Table of Common JavaScript Libraries
Library | Usage | Best For |
---|---|---|
jQuery | DOM manipulation, animations | Simple projects, rapid prototyping |
React | Component-based UI development | Dynamic, state-driven web apps |
Vue.js | Progressive framework for building UIs | Small to medium projects with complex interactions |
Optimizing Website Performance Using JavaScript Tools
Website performance is crucial for user experience and SEO ranking. JavaScript provides a wide range of tools and techniques to improve loading times, interactivity, and overall performance. By leveraging these tools, developers can optimize client-side operations, reduce render times, and ensure smooth interactions.
Some of the most effective JavaScript-based performance enhancements come from optimizing resource loading, minimizing JavaScript execution time, and using asynchronous processes. Implementing the right tools can significantly boost efficiency, leading to faster, more responsive websites.
Key JavaScript Tools for Performance Optimization
- Lighthouse - A powerful tool for auditing performance, accessibility, and best practices. It provides actionable insights and suggestions for optimization.
- Webpack - A module bundler that helps optimize loading times by bundling JavaScript files and reducing unnecessary code.
- Rollup - A JavaScript module bundler that performs tree-shaking to eliminate dead code and improve the final bundle size.
- Parcel - A zero-config bundler that automatically optimizes assets, offering fast build times and easy integration with modern JavaScript features.
Important: Always prioritize asynchronous loading for non-essential scripts to avoid blocking the critical rendering path.
Performance Optimization Techniques
- Lazy Loading - Delaying the loading of off-screen or non-critical resources until they are needed. This technique ensures that the initial page load is faster.
- Code Splitting - Dividing the JavaScript code into smaller chunks that are loaded only when necessary, reducing the initial bundle size.
- Minification - Removing unnecessary characters from JavaScript files (such as spaces, comments, and line breaks) to reduce file size.
- Service Workers - Using service workers to cache assets and improve loading speeds on subsequent visits.
Performance Metrics and Monitoring
Monitoring and measuring the impact of optimizations is critical to ensuring ongoing performance improvements. Below are common tools for tracking performance:
Tool | Use |
---|---|
Google Analytics | Track user interactions and loading times to identify potential bottlenecks. |
WebPageTest | Provide detailed insights into website load times from different locations and devices. |
Chrome DevTools | Offer in-depth analysis of network performance, resource loading, and JavaScript execution. |
Integrating External APIs for Dynamic Content in Web Development
Integrating external APIs into a website is essential for delivering real-time data and enhancing user interactivity. With JavaScript, developers can easily fetch data from external servers and display it dynamically without reloading the entire page. This approach is crucial for improving the overall user experience, making the website feel more responsive and engaging. The power of APIs lies in their ability to provide content such as weather updates, social media feeds, and user-generated data on demand.
Using JavaScript, APIs are typically accessed via HTTP requests. The most common methods for fetching data are GET and POST requests, which can be made using the built-in `fetch()` function or libraries like Axios. Once the data is received, JavaScript manipulates the DOM to present the content dynamically, ensuring the page remains interactive and fast.
Methods for API Integration
There are several strategies for integrating APIs, depending on the complexity and needs of the website:
- REST APIs: The most widely used method for accessing data. REST APIs use standard HTTP requests and return data in JSON format, making it easy for JavaScript to parse and display it.
- WebSockets: Used for real-time communication, WebSockets provide a persistent connection, allowing the server to send data to the client as soon as it is available.
- GraphQL: A more flexible approach than REST, where clients can query exactly the data they need, improving efficiency and reducing over-fetching.
Example API Integration
The following is an example of how JavaScript can fetch data from an API and dynamically display it on the webpage:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
document.getElementById('content').innerHTML = data.result;
})
.catch(error => console.log('Error:', error));
Considerations for API Integration
When integrating APIs, developers should be mindful of several factors:
- Data Handling: Always ensure proper handling of the data, especially when working with large amounts of it.
- API Rate Limits: Many APIs impose limits on how often data can be requested. Exceeding these limits can lead to temporary or permanent bans.
- Security: Always use secure methods to handle sensitive data, such as API keys, and ensure that the API supports HTTPS to prevent data breaches.
Important Note
API integration is a powerful tool for enhancing website functionality, but it must be done responsibly to avoid performance issues and data security risks.
Example of API Data Display in Table
Data Type | Value |
---|---|
Temperature | 22°C |
Humidity | 56% |
Building Adaptive Websites with JavaScript and CSS Frameworks
When designing websites that adjust to various screen sizes, utilizing JavaScript and CSS frameworks can drastically simplify the process. These frameworks come with pre-built components that help developers implement responsive designs quickly. By integrating both JavaScript functionalities and CSS styling tools, developers can create websites that perform efficiently across different devices, ensuring a seamless user experience.
CSS frameworks, such as Bootstrap or Foundation, provide a foundation of grid systems, typography, and customizable elements, enabling developers to create fluid layouts. On the other hand, JavaScript adds dynamic interactivity, like real-time data updates or complex animations, enhancing the overall responsiveness of the site.
Key Aspects of Responsive Web Design
- Fluid Grids: A grid system that adjusts the layout based on the screen width.
- Media Queries: CSS rules that apply specific styles depending on the device's characteristics (e.g., screen size or orientation).
- Flexible Images: Images that resize proportionally according to the screen resolution.
- JavaScript Enhancements: Dynamic content loading and interactive elements tailored to different screen sizes.
Responsive design ensures that websites adapt efficiently to the viewer’s device, whether it’s a smartphone, tablet, or desktop. This approach leads to improved performance and user satisfaction.
Frameworks to Consider
- Bootstrap: A widely used CSS framework that provides a mobile-first grid system and responsive utilities.
- Foundation: Another popular framework, offering flexible grids, customizable features, and advanced responsive techniques.
- Tailwind CSS: A utility-first framework known for its customizable and rapid styling features.
- Vue.js/React.js: JavaScript frameworks that, when combined with CSS frameworks, can build highly responsive, data-driven websites.
Example of Grid Layout in a Framework
Element | Grid Size | Media Query |
---|---|---|
Header | 12 columns | All screen sizes |
Sidebar | 4 columns | ≥ 768px |
Main Content | 8 columns | ≥ 768px |
Using JavaScript for Real-time Data Updates in Websites
Real-time data updates on websites are becoming increasingly essential, especially for platforms that rely on live user interactions, such as social media, online trading, and collaborative tools. JavaScript provides powerful techniques to handle real-time data efficiently, enabling smooth user experiences. With the help of APIs, WebSockets, and frameworks like Node.js, developers can push data changes directly to the user's browser without needing to refresh the page.
JavaScript can continuously fetch and display new data, allowing websites to remain interactive and dynamic. This ability to update the content instantly enhances user engagement and reduces latency. Several tools and approaches make this possible, including polling mechanisms, server-sent events (SSE), and the WebSocket protocol.
Key Techniques for Real-time Data Updates
- WebSockets: Establish a two-way communication channel between the server and the client for instant data transmission.
- Server-Sent Events (SSE): Allow the server to push updates to the client over a single HTTP connection.
- Polling: Frequently request data from the server at regular intervals, updating the user interface with fresh information.
WebSockets offer the most efficient method for real-time communication, as they maintain an open connection, allowing the server to send data immediately when it becomes available.
Example: Real-time Stock Prices
Below is an example of a simple table displaying stock prices updated in real-time:
Stock | Price | Change |
---|---|---|
Apple | $145.67 | +0.5% |
$2729.45 | -0.3% | |
Amazon | $3342.12 | +1.2% |
Using WebSockets, this table could be dynamically updated as the stock prices change without requiring the user to refresh the page.
Ensuring Optimal User Experience Through Effective Debugging and Testing in JavaScript
Debugging and testing are vital steps in web development to ensure that your JavaScript code runs seamlessly. These processes help identify and resolve issues that may arise during execution, improving both functionality and user satisfaction. Proper debugging tools and testing frameworks are essential in detecting errors before they affect the end user.
By utilizing advanced debugging techniques and performing thorough testing, developers can prevent bugs from disrupting the user experience. With tools like browser developer tools, unit testing frameworks, and integration tests, you can ensure your website behaves as intended across various environments.
Debugging Strategies to Improve Code Quality
Debugging in JavaScript involves a systematic approach to locate and fix issues. The following techniques are commonly used to ensure smooth code execution:
- Console Logging: Use
console.log()
to output variable values and track execution flow. - Breakpoints: Set breakpoints in browser developer tools to pause code execution at specific points for inspection.
- Error Handling: Implement
try...catch
blocks to catch exceptions and handle errors gracefully.
Effective debugging helps identify unexpected behavior in code, ensuring users do not encounter issues during their interactions with your website.
Testing Practices for Robust JavaScript Code
Testing is an essential part of the development process to verify that your JavaScript code works correctly across different scenarios. The following methods can enhance the quality of your testing:
- Unit Testing: Focus on testing individual functions or methods using frameworks like Jest or Mocha.
- Integration Testing: Verify that different parts of the system work together as expected.
- End-to-End Testing: Simulate real user interactions to ensure that the entire system functions properly in a live environment.
Additionally, automated testing tools like Selenium or Cypress can speed up the testing process by simulating user actions and checking for regressions.
Key Metrics to Track During Testing
Metric | Purpose |
---|---|
Test Coverage | Ensures that most of the code is being tested, reducing the risk of untested areas causing problems. |
Error Rate | Tracks the number of errors during testing to identify problematic sections in the code. |
Response Time | Measures how quickly the system responds to user actions, directly affecting user experience. |
Tracking these metrics ensures that your JavaScript code is optimized for performance, ensuring a seamless user experience.
Deploying Your JavaScript-based Website to Production
After completing your JavaScript-driven website, the next critical step is deployment. Deployment refers to moving your local website files to a production environment, making it accessible to users via the web. This process involves several stages, from preparing your website for live environments to selecting the right hosting services. Proper deployment ensures smooth performance, security, and scalability of your website.
Choosing a hosting provider, configuring domain names, and managing server resources are just a few components of this process. It's essential to be mindful of aspects such as error handling, performance optimization, and security configurations when deploying a JavaScript website.
Steps to Deploy Your Website
- Build the Production Version: Before deployment, use build tools like Webpack or Parcel to bundle and minify your JavaScript files. This reduces load times and optimizes performance.
- Select a Hosting Provider: Choose between traditional hosting (like Apache or Nginx) or cloud-based solutions (like AWS, Netlify, or Vercel) based on your website's needs.
- Upload Files: After setting up your hosting environment, upload the static files (HTML, CSS, JavaScript) to the server.
- Set Up a Domain Name: Point your domain to the hosting server to ensure that users can access your site using a custom URL.
- Test the Deployment: Thoroughly test your website in a live environment to ensure everything functions as expected.
Common Hosting Platforms for JavaScript Websites
Provider | Type | Best For |
---|---|---|
AWS | Cloud Hosting | Scalable and customizable hosting solutions for large-scale projects |
Netlify | Static Hosting | Fast and simple hosting for static sites with automatic builds |
Vercel | Cloud Hosting | Optimized for Next.js apps and fast deployment |
Important: Ensure your website is fully tested in a staging environment before deploying to production to avoid potential issues.