Can You Make a Website with C++
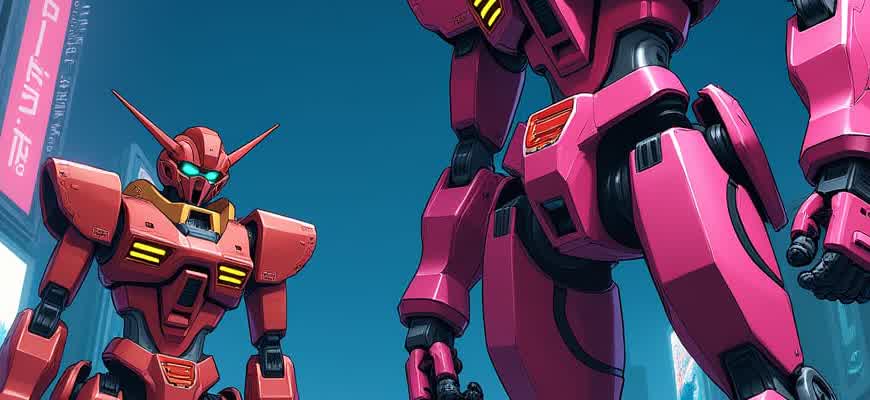
While C++ is primarily known for system-level programming, games, and performance-critical applications, it is not typically used for web development. However, some developers may wonder whether it’s possible to use C++ for creating websites or web applications. The short answer is: yes, but with limitations and complexities.
In web development, traditional tools like HTML, CSS, and JavaScript dominate the landscape. C++, on the other hand, lacks the built-in libraries and frameworks for easy integration into front-end or back-end workflows. Despite this, C++ can still play a role in web technologies in specific cases. Here’s how:
- Server-Side Performance: C++ can be used to develop high-performance server-side applications, such as web APIs.
- CGI Programming: Common Gateway Interface (CGI) allows C++ to generate dynamic web content, though it’s not as efficient as modern solutions.
- WebAssembly: This allows C++ code to run in the browser, providing enhanced performance for web applications.
However, using C++ for web development comes with challenges, such as:
- Steeper learning curve compared to more web-friendly languages.
- Limited support for modern front-end features like responsive design and interactivity.
- Additional complexity in deployment and integration with existing web technologies.
While C++ is not the ideal choice for most web development tasks, it can be leveraged in niche scenarios where performance is critical.
To summarize, C++ is not the go-to option for typical website creation, but its role in the server-side or performance-critical components of a web project should not be dismissed.
Approach | Benefits | Drawbacks |
---|---|---|
Server-Side with C++ | High performance, low-level control | Complex setup, limited libraries |
CGI | Dynamic content generation | Old technology, inefficient |
WebAssembly | Performance in browsers | Requires additional tools, not universally supported |
Understanding the Role of C++ in Web Development
C++ is not commonly associated with web development, but it does have specific uses that make it relevant for certain parts of the web ecosystem. Although languages like JavaScript, Python, and PHP dominate the landscape of server-side and client-side development, C++ can be employed in web development in scenarios that require high performance and direct hardware control. When optimized for specific tasks, C++ can offer speed advantages that other languages cannot match.
In web development, C++ is typically used in performance-critical components, such as backend services, web servers, or browser engines. It is also used to create APIs or plugins that can be integrated into web applications to handle complex computations or real-time data processing. Additionally, C++ plays a key role in the development of embedded systems and applications that interact with web-based services.
Key Areas Where C++ Contributes to Web Development
- Web Servers: C++ can be used to build high-performance web servers capable of handling large volumes of requests efficiently.
- Browser Engines: Many modern browsers (e.g., Google Chrome, Mozilla Firefox) are built on C++ code for rendering web pages quickly.
- API Development: C++ can be used to create fast and efficient APIs that interact with other web technologies, enhancing the overall performance of a web application.
Advantages of Using C++ in Web Development
- High Performance: C++ offers unmatched speed and control over system resources, making it ideal for performance-intensive tasks.
- System-Level Access: C++ allows developers to write low-level code, providing direct access to memory and hardware, which is beneficial for certain web applications.
- Scalability: C++ can be optimized to handle large-scale systems and manage concurrent processes more efficiently than many other programming languages.
Limitations and Considerations
C++ is a complex language that requires careful management of memory and resources. It may not be the best choice for projects where development speed and ease of use are prioritized over performance.
Technology | Use in Web Development | Performance |
---|---|---|
C++ | Web Servers, Browser Engines, APIs | High |
JavaScript | Client-Side Scripting | Moderate |
Python | Server-Side Scripting | Moderate |
Advantages and Disadvantages of Using C++ for Website Development
C++ is a powerful programming language traditionally used for system software, game development, and performance-critical applications. However, with the rise of web technologies, developers have started to explore its potential in building websites. While it offers some notable benefits, there are challenges that come with using C++ for web development.
In this article, we will examine the key advantages and disadvantages of using C++ for creating websites. These factors play a significant role in determining whether it's a suitable choice for a given project.
Advantages of C++ for Websites
- Performance: C++ is known for its high execution speed and efficient memory management, which can be particularly useful for websites requiring intensive calculations, real-time processing, or handling large amounts of data.
- Low-Level Control: C++ provides granular control over hardware resources, which can be beneficial when optimizing web applications that require fine-tuned resource management, such as embedded systems or custom server environments.
- Wide Range of Libraries: The language has access to a vast ecosystem of libraries and frameworks that can assist in building complex web applications with specific functionality.
Disadvantages of C++ for Websites
- Complexity: C++ is a more complex language compared to web-centric languages like JavaScript or Python. Its steep learning curve can slow down development and increase the chances of introducing bugs.
- Development Time: Writing code in C++ generally takes more time and effort, which can increase the overall project timeline. Moreover, the need for manual memory management further complicates the process.
- Lack of Web-Focused Tools: Unlike languages designed specifically for web development (e.g., PHP, Ruby), C++ does not have extensive built-in libraries or tools for creating web interfaces, making the process less efficient.
C++ is great for performance-intensive applications, but it may not be the most practical choice for standard web development needs where rapid prototyping and ease of use are key.
Comparing C++ with Other Web Development Languages
Feature | C++ | JavaScript | Python |
---|---|---|---|
Performance | High | Medium | Medium |
Development Speed | Slow | Fast | Fast |
Memory Management | Manual | Automatic | Automatic |
Tooling | Limited | Extensive | Good |
Setting Up a C++ Environment for Web Development
Developing web applications using C++ requires setting up the right tools and libraries. While C++ is traditionally used for system-level programming, it can be leveraged for web development by utilizing frameworks and proper configurations. The process of setting up a C++ development environment for web projects involves installing compilers, web frameworks, and configuring necessary services for seamless development.
This guide will walk you through the key steps involved in setting up a C++ development environment, including required software, libraries, and best practices for working with web projects in C++.
Key Components for a C++ Web Development Setup
- C++ Compiler: A compiler is necessary to build your C++ applications. Popular choices include GCC for Linux and Clang for macOS. For Windows, MinGW or Visual Studio can be used.
- Web Frameworks: C++ does not have native support for web development, so using a framework like C++ REST SDK or CppCMS is essential. These frameworks provide the necessary tools to handle HTTP requests, responses, and session management.
- Web Server: You will need a web server to host your application. Common choices for C++ web applications are Apache with CGI or nginx for reverse proxying requests.
Step-by-Step Setup Process
- Install a C++ Compiler: First, you need to install a suitable C++ compiler for your system.
- Choose a Web Framework: Depending on your project, select a framework like CppCMS or C++ REST SDK to handle web requests and responses.
- Install Dependencies: Install the required libraries and dependencies for the framework you selected. This could include installing libraries for JSON parsing, HTTP handling, or database connections.
- Set Up a Web Server: Install and configure a web server such as Apache or nginx to serve your application. Set up necessary configurations to forward requests to your C++ backend.
Note: Make sure your server is correctly configured to handle requests from the framework and provide necessary security features such as SSL/TLS support.
Recommended Tools and Libraries
Tool/Library | Description |
---|---|
CppCMS | A web development framework specifically designed for C++. Provides tools for building dynamic websites and handling high-traffic applications. |
C++ REST SDK | A powerful library for building HTTP-based services in C++. Ideal for RESTful APIs and communication over HTTP. |
Boost Libraries | A collection of portable C++ source libraries that provide additional functionality for C++ web projects, including threading, networking, and file I/O. |
Combining C++ with Front-End Web Technologies
Integrating C++ into web development usually requires interaction with other technologies like HTML, CSS, and JavaScript. While C++ is not natively designed for web development, it can still play a significant role in server-side processing or performance-critical tasks. To connect C++ with front-end technologies, developers typically rely on WebAssembly (Wasm) or server-side frameworks that bridge the gap between C++ code and client-side interactions.
When integrating C++ with HTML, CSS, and JavaScript, the most common approach involves compiling C++ into WebAssembly. This allows C++ code to run in the browser, enabling high-performance computations while interacting with HTML, CSS, and JavaScript. By doing so, C++ logic can be embedded in web pages, enhancing their functionality and speed.
How C++ Interacts with Web Technologies
- WebAssembly (Wasm): A low-level bytecode format that allows C++ code to run in the browser, alongside JavaScript and other web technologies.
- Server-Side Processing: C++ can handle heavy computations on the server, sending the results to the client using JavaScript.
- API Communication: C++ applications can communicate with front-end web pages via APIs, often through RESTful services or WebSocket connections.
"C++ can provide performance benefits by handling complex logic, while JavaScript ensures smooth user interaction and dynamic content generation."
Implementation Example
Step | Action |
---|---|
1 | Compile C++ code into WebAssembly using tools like Emscripten. |
2 | Load the Wasm module in the HTML file using JavaScript. |
3 | Use JavaScript to call C++ functions and pass data between the client and the server. |
Tip: Combining C++ with WebAssembly significantly boosts performance in web applications that require intensive calculations, such as games or simulations.
Using C++ Frameworks for Web Development: Is It Worth It?
While C++ is a powerful language known for its performance, it is not traditionally associated with web development. However, there are several C++ frameworks designed to facilitate the creation of web applications. These frameworks aim to combine the speed and efficiency of C++ with the needs of modern web services. But the question remains: is it truly practical to use C++ for web development compared to more popular languages like JavaScript or Python?
In the realm of web development, there are distinct pros and cons to consider when opting for C++ frameworks. They can deliver impressive performance, especially for high-load applications, but come with certain complexities in terms of development speed and ecosystem support. Below is an overview of key factors to consider:
Pros and Cons of Using C++ for Web Development
Performance: C++ excels in providing high-performance solutions, especially when handling large-scale applications that require minimal latency.
- Performance: C++ frameworks are ideal for performance-critical applications where low-level system access is necessary.
- Concurrency: C++ supports advanced threading models, which can be beneficial for web applications that need to handle multiple requests simultaneously.
- Memory Management: Fine-grained control over memory allows developers to optimize resource usage and improve the efficiency of applications.
Challenges of Using C++ for Web Development
Complexity: C++ frameworks tend to be more complicated and require a deeper understanding of low-level programming concepts compared to higher-level languages.
- Steeper Learning Curve: Developers must have a solid grasp of both the C++ language and its associated frameworks.
- Development Speed: Compared to more web-friendly languages, C++ can significantly slow down the development process due to its low-level nature.
- Limited Ecosystem: While there are some notable C++ frameworks, the overall ecosystem and community support is less robust compared to languages like JavaScript or Ruby.
Notable C++ Web Frameworks
Framework | Key Features | Use Cases |
---|---|---|
CppCMS | High performance, asynchronous processing | Real-time web apps, high-performance websites |
Poco | Cross-platform, libraries for network and database access | Web servers, cloud applications |
Wt | Widget-based web development, client-server model | Interactive web apps, user interfaces |
While C++ frameworks can provide unmatched performance, they are not always the best choice for standard web applications. Developers should carefully consider their project’s needs and the trade-offs involved before deciding to use C++ for web development.
Handling Server-Side Operations with C++ for Web Applications
Using C++ for server-side operations in web applications offers a high level of control over performance and resource management. Although C++ is not traditionally associated with web development, it can be effectively used to handle server-side logic, especially for performance-critical applications. The language allows developers to fine-tune processes, minimize overhead, and optimize response times, making it suitable for scenarios requiring high throughput or low-latency operations.
One of the challenges of using C++ for web servers is managing HTTP requests and responses, as C++ does not have built-in libraries like higher-level languages. However, frameworks like CppCMS or Poco can help bridge this gap, offering features such as URL routing, request parsing, and integration with databases. These tools can facilitate the creation of web applications where C++ handles critical server operations.
Key Concepts for C++ in Server-Side Development
- Concurrency and Multithreading: C++ provides low-level control over threads, which is crucial for handling multiple simultaneous requests efficiently.
- Memory Management: With manual memory management, C++ allows developers to optimize how memory is allocated and deallocated, a key consideration for high-performance servers.
- Integration with Databases: C++ can be used to interact with databases using libraries such as MySQL Connector or ODBC.
Example of server-side flow:
- The client sends an HTTP request to the server.
- The server processes the request using C++ code, which may involve database queries, business logic, and data manipulation.
- The server generates an HTTP response, which is sent back to the client.
Advantages and Disadvantages
Advantages | Disadvantages |
---|---|
High performance and low latency due to fine-grained control. | Requires more development time compared to high-level languages. |
Ability to handle resource-intensive operations efficiently. | Lack of native web frameworks and tools compared to other languages. |
Manual memory management for optimization. | Can be more error-prone due to manual memory and thread management. |
Note: While C++ offers superior performance, it is important to evaluate the trade-offs between development speed and system performance when choosing it for web applications.
Security Concerns When Building Websites with C++
Developing websites using C++ introduces unique security challenges that developers need to be aware of. While C++ offers significant performance benefits, it requires more attention to low-level details that are handled automatically by higher-level languages. This often leads to increased risk if proper precautions are not taken. Vulnerabilities such as memory management errors, improper input validation, and insufficient encryption can result in critical security breaches.
When choosing C++ for web development, the responsibility of managing security rests heavily on the developer. Unlike modern web frameworks that provide built-in security features, C++ requires manual implementation of secure practices. Below are some of the key security concerns to consider when building websites with C++.
Common Security Issues
- Buffer Overflows: C++ is prone to buffer overflow vulnerabilities due to direct memory access. These can allow attackers to execute arbitrary code or crash the server.
- Memory Leaks: Improper memory management can cause memory leaks, which may lead to resource exhaustion, affecting website performance or availability.
- Unvalidated Input: Websites written in C++ often suffer from insufficient input validation, which can lead to injection attacks such as SQL injections or cross-site scripting (XSS).
Best Practices for Mitigating Security Risks
- Use Secure Libraries: Leverage established, well-tested libraries for encryption, authentication, and session management. This reduces the chance of introducing vulnerabilities.
- Regularly Update Dependencies: Ensure that any external libraries or dependencies are regularly updated to fix known security flaws.
- Implement Proper Input Sanitization: Always sanitize user input to prevent malicious data from being executed by the web server.
Security Measures Table
Security Concern | Mitigation Strategy |
---|---|
Buffer Overflow | Use safe string handling functions, such as std::string or bounded functions like strncpy . |
Memory Leak | Employ smart pointers or garbage collection libraries to ensure proper memory management. |
Unvalidated Input | Implement comprehensive input validation and sanitize inputs to prevent injection attacks. |
Developers must remain vigilant in implementing security features manually in C++, as this language lacks the built-in protections offered by other modern web development frameworks.
Real-World Examples of Websites Built Using C++
While C++ is typically associated with systems programming and applications requiring high performance, it can also be used to develop web technologies and frameworks. Though not as common as languages like JavaScript or Python for web development, C++ can still play a significant role in powering websites and web applications. Websites that require high-speed processing or deal with complex computations often leverage the efficiency and power of C++ to handle backend tasks or optimization.
Several well-known platforms have used C++ to build and enhance parts of their infrastructure, particularly for performance-heavy services. Below are some examples of how C++ has been employed in real-world web development.
Examples of Websites Using C++
- Facebook - The social media giant uses C++ in its backend systems to optimize performance in areas like the database and server-side computations.
- Instagram - Similar to Facebook, Instagram utilizes C++ for various backend components, especially for image and video processing algorithms.
- Google - Google uses C++ for services like Search and YouTube, especially in components requiring fast processing of large amounts of data.
Why C++ is Chosen for Web Development
C++'s role in web development is largely centered around its ability to manage high-performance tasks, such as video streaming, gaming, and real-time processing. Some reasons developers might choose C++ include:
- Speed and Efficiency - C++ is known for its ability to process tasks quickly, making it ideal for backend operations involving large datasets or real-time applications.
- Memory Management - With its direct control over system memory, C++ allows for optimized handling of resources, which is critical for complex systems.
- Cross-Platform Capabilities - C++ can be used across different operating systems, offering flexibility for building scalable web applications.
Important Considerations
While C++ offers many advantages, it requires more expertise compared to higher-level languages typically used in web development. Developers must also deal with potential complexities such as memory leaks or manual resource management, which can complicate the development process.
Performance Optimization with C++
Task | Reason for C++ Usage |
---|---|
Real-time Analytics | Fast data processing and analysis without delays |
Large-scale Data Handling | Efficient memory management and handling of massive datasets |
Complex Calculations | High computational performance required for complex algorithms |