Next Js Drag and Drop Website Builder
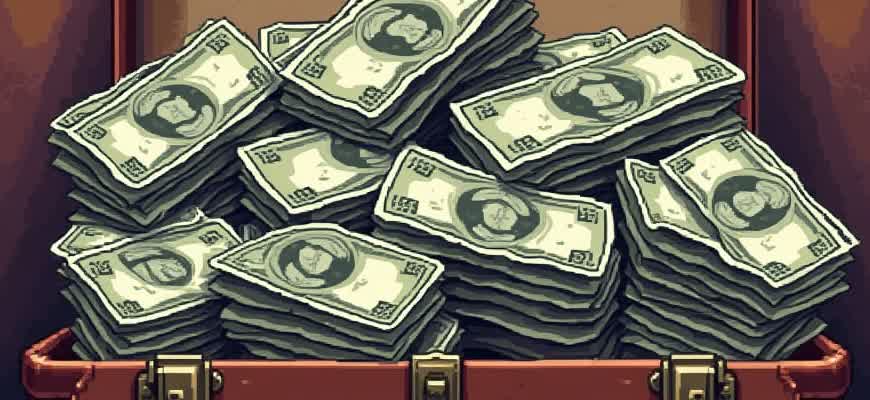
Building websites with drag-and-drop functionality has become a standard for creating user-friendly and interactive web applications. With the growing demand for fast and efficient web development tools, Next.js has emerged as a robust framework for creating highly customizable website builders. Leveraging its server-side rendering capabilities, developers can create dynamic and responsive websites without sacrificing performance.
Key Features of a Next.js Drag-and-Drop Builder:
- Easy-to-use interface with minimal setup
- Real-time updates and preview of changes
- Customizable components for flexibility
- SEO-friendly architecture for better search engine ranking
Advantages:
- Enhanced performance due to static site generation and server-side rendering
- Scalability and ease of maintenance with modular architecture
- Faster development process with pre-built components and layouts
"Next.js enables developers to focus on building custom user experiences while handling the complexities of performance optimization and server-side rendering."
Comparison with Traditional Website Builders:
Feature | Next.js Builder | Traditional Builder |
---|---|---|
Performance | Optimized with SSR & SSG | Limited to client-side rendering |
Flexibility | Highly customizable with React components | Predefined templates with limited customization |
SEO | SEO-optimized out-of-the-box | Requires additional plugins |
Integrating Custom Components into Your Next.js Project
Building a dynamic website with Next.js involves integrating various reusable components. Custom components are essential to provide specific functionality or a unique user experience. Next.js, being a highly extensible framework, makes it easier to seamlessly integrate these components into your application, whether you're developing a simple landing page or a complex drag-and-drop builder.
By creating custom components, you can break your project into manageable, reusable pieces, making development faster and easier. These components can be added to your project through local imports, allowing flexibility and control. Additionally, by using Next.js's server-side rendering and static site generation, custom components can be efficiently optimized for performance and SEO.
Steps to Integrate Custom Components
- Define your custom component: Create a new component file in the components directory.
- Import the component: Use the
import
statement to include the custom component into your pages. - Pass props: Pass necessary data or functionality to the component using props.
- Use hooks (if necessary): Integrate React hooks to manage state or side effects within your component.
Custom components provide better organization and scalability to your Next.js projects, allowing you to focus on reusable building blocks that improve code maintainability.
Example Code Snippet
import MyComponent from '../components/MyComponent';
const Page = () => {
return (
);
};
export default Page;
Handling External Libraries and Frameworks
When integrating third-party libraries or external frameworks with custom components, you should follow a structured approach:
- Install dependencies: Use
npm
oryarn
to install any necessary libraries. - Import external libraries: Include the library at the top of your component file.
- Integrate with existing component logic: Ensure external libraries work seamlessly within the component’s lifecycle.
Tips for Efficient Component Integration
Tip | Description |
---|---|
Lazy Loading | Use dynamic imports to load heavy components only when necessary to improve performance. |
Code Splitting | Split components into smaller bundles for better load times, especially in larger applications. |
Optimizing Performance in a Next.js Drag and Drop Website Builder
When building a drag-and-drop website builder with Next.js, performance optimization is crucial to ensure smooth user interaction and fast load times. The application must handle dynamic content efficiently while minimizing unnecessary rendering and excessive re-renders. By following best practices, it’s possible to maintain high performance while delivering a rich and responsive user experience.
There are several strategies that can be employed to enhance the performance of a drag-and-drop interface, from lazy loading to effective state management. Below are key approaches to optimize the performance of such applications.
Key Performance Optimization Techniques
- Lazy Loading: Load components only when they are needed. This reduces the initial load time and ensures faster page rendering.
- Code Splitting: Split the code into smaller bundles to reduce the size of the initial JavaScript payload.
- Memoization: Use React’s
useMemo
andReact.memo
to avoid unnecessary re-renders of components that haven’t changed. - Virtualization: Render only the visible parts of the builder’s interface, especially when dealing with long lists or complex structures.
"Effective state management and efficient data flow are key to keeping the application fast and responsive."
Optimizing Drag-and-Drop Interactions
- Debouncing Events: Apply debouncing to minimize the number of updates during drag-and-drop events, which can be taxing on the system.
- Optimized Re-renders: Implement conditional rendering so that components only update when necessary. Using techniques like shallow comparison and memoization helps reduce the rendering overhead.
- Event Throttling: Limit the frequency of event handlers during drag events to avoid overloading the main thread and causing jank.
Performance Benchmarks and Monitoring
Regularly measure the performance of your Next.js drag-and-drop builder using browser tools and performance profiling libraries. Below is an example table showcasing common metrics to monitor:
Metric | Description | Ideal Value |
---|---|---|
First Contentful Paint (FCP) | Time taken for the first element to appear on the screen | Under 1 second |
Time to Interactive (TTI) | Time taken for the page to become fully interactive | Under 3 seconds |
Jank | Frame rate drops during drag-and-drop actions | Below 100ms |
How to Implement Advanced Styling in Your Next.js Website Builder
Advanced styling in a Next.js drag-and-drop website builder allows users to create custom layouts and designs without coding. By integrating various CSS methodologies, you can provide a more flexible and dynamic styling experience. This approach also ensures better scalability and maintainability of styles as your application grows.
To effectively implement advanced styling in your Next.js builder, consider using CSS-in-JS solutions like styled-components or Emotion, which offer greater control over styling and are compatible with Next.js. Additionally, leveraging CSS grid and flexbox will give you more precise control over layout, allowing the user to position elements more intuitively in the drag-and-drop interface.
Using Styled Components for Advanced Customization
Styled-components allow you to define styles directly within your React components, making it easier to manage dynamic styling in a modular way. Here’s how to integrate it into your project:
- Install styled-components: npm install styled-components
- Import it into your component: import styled from 'styled-components'
- Create a styled component: const Button = styled.button`background: ${props => props.bgColor};`
- Use the styled component in your JSX:
By using styled-components, you can dynamically adjust styles based on user input or predefined themes, creating a responsive and customizable design environment for users.
Leveraging Flexbox and Grid Layouts for Precision
Next.js allows seamless integration of flexbox and CSS grid, which are crucial for achieving flexible layouts in your builder. These tools help ensure that elements are properly aligned, even when content size changes dynamically.
Flexbox is ideal for one-dimensional layouts (rows or columns), while CSS grid is perfect for two-dimensional layouts (both rows and columns).
Layout Type | Use Case |
---|---|
Flexbox | When you need elements aligned in a single row or column, and need flexibility with spacing. |
Grid | For complex, multi-row and multi-column layouts with precise control over item positioning. |
Integrating both Flexbox and CSS grid into your website builder will enhance user experience by providing customizable layout options that adapt to different screen sizes.
Adding Dynamic Content with Next.js Drag and Drop Features
Next.js offers a powerful way to integrate drag and drop functionality for building dynamic websites. By leveraging this feature, users can easily customize their websites with flexible content placement. Dynamic content is a key aspect of modern websites, allowing for interactive user experiences and real-time updates without the need for page reloads. With the drag-and-drop capabilities in Next.js, developers can create a seamless interface for users to rearrange and interact with elements on the page.
To implement dynamic content, Next.js makes it simple to manage state and handle user interactions. The drag-and-drop functionality is powered by React, which is fully supported by Next.js, and it can be easily customized for different use cases. For instance, users can add or remove components like images, text blocks, or even interactive widgets. This flexibility makes it possible to create highly dynamic pages where content can be personalized on the fly.
Using Drag and Drop with Next.js
One of the key benefits of integrating drag-and-drop features into a Next.js website is the ability to handle content dynamically without reloading the page. Below are some important points on how to implement and manage dynamic content:
- State Management: Use React state to track the position and data of draggable elements.
- Client-Side Updates: Ensure that updates happen on the client side to prevent unnecessary server requests and to improve user experience.
- Responsive Design: Implement a responsive layout so that the drag-and-drop interface works across different devices.
With drag-and-drop features, developers can offer a more intuitive interface for users to arrange and modify their content. This boosts engagement and enhances the user experience.
Integrating Dynamic Components
When building dynamic content blocks, it's important to consider how the content will be structured. Here's a table that outlines the common dynamic components you can include in a Next.js drag-and-drop interface:
Component | Functionality |
---|---|
Image Gallery | Allows users to add, rearrange, and remove images dynamically. |
Text Block | Enables users to add, edit, and reposition text content with ease. |
Video Player | Drag and drop to insert and manage video content on the page. |
By utilizing these components and managing state effectively, developers can build robust and interactive websites with minimal complexity.
Debugging Common Issues in Next.js Website Builder
When building websites with Next.js, developers often encounter common issues related to drag-and-drop functionality. Debugging these problems effectively is crucial to ensuring a smooth user experience and functionality. This article addresses common errors and provides practical solutions to resolve them.
In a Next.js-based website builder, issues often stem from improper state management, component re-rendering problems, or third-party library incompatibilities. By identifying the root causes of these problems, developers can streamline the debugging process and enhance the performance of their projects.
1. State Management Issues
One of the most frequent issues in a drag-and-drop interface is improper handling of component state. The drag-and-drop functionality relies heavily on managing the position of elements dynamically. If state updates are not properly handled, elements may not move as expected, or the UI may freeze.
Tip: Ensure that the state changes are made immutably and that they trigger re-renders when necessary.
- Check for unnecessary re-renders caused by improper state dependencies.
- Use useEffect or useMemo hooks for handling state updates efficiently.
- Consider using Redux or Zustand for managing complex state changes in larger projects.
2. Component Re-Rendering Problems
Excessive re-renders can degrade the performance of a drag-and-drop interface, making the interaction feel sluggish. This typically occurs when components are being re-rendered unnecessarily after each drag or drop event.
Tip: Use React’s memoization techniques, such as React.memo and useCallback, to prevent unnecessary re-renders.
- Identify components that do not need to re-render on every drag event.
- Implement React.memo to avoid re-renders of static components.
- Optimize event handlers using useCallback to avoid creating new instances on each render.
3. Compatibility with Third-Party Libraries
Integrating third-party libraries can introduce issues if the library isn’t fully compatible with Next.js. This might lead to unexpected behaviors, such as improper rendering of draggable components or conflicts with Next.js’s server-side rendering.
Tip: Always check the library’s documentation for Next.js compatibility or consider using alternatives.
Library | Issue | Solution |
---|---|---|
react-dnd | Fails to render correctly with SSR | Use dynamic imports with { ssr: false } to load the library only on the client side. |
react-beautiful-dnd | Conflicts with Next.js routing | Ensure correct implementation of the DragDropContext component and review routing logic. |
Best Practices for Deploying Your Next.js Drag-and-Drop Website
Deploying a Next.js drag-and-drop website requires careful planning to ensure that the site performs optimally in production. The build process, server configuration, and user experience must all be streamlined for fast loading times and reliability. Below are some essential best practices to keep in mind during deployment.
To begin with, leveraging a serverless environment for hosting can significantly improve scalability and reduce operational overhead. Ensure that your drag-and-drop functionalities are optimized, especially when handling large files or dynamic content. This includes implementing proper caching strategies and using CDN for static assets.
Key Deployment Considerations
- Optimize Static File Delivery: Use a CDN to deliver static assets like images, CSS, and JavaScript files. This reduces load times and improves user experience.
- Utilize Serverless Functions: Serverless functions are perfect for handling dynamic content in your drag-and-drop site without the need to manage traditional servers.
- Ensure Responsive Design: Make sure your website adapts to various screen sizes, especially since drag-and-drop builders require a flexible UI for mobile users.
- Monitor Performance: Use tools like Lighthouse or Web Vitals to track the performance of your Next.js app post-deployment and optimize areas of improvement.
Common Deployment Pitfalls to Avoid
- Failing to Optimize Images: Unoptimized images can slow down page load times. Use formats like WebP and implement lazy loading.
- Ignoring Caching: Not caching API responses or static content can cause unnecessary server requests, impacting site speed.
- Not Configuring Error Handling: Ensure that your site gracefully handles errors such as missing assets or failed API requests.
Deploying a drag-and-drop builder can be complex, but paying attention to these details ensures that your site performs at its best and provides a seamless experience for users.
Recommended Hosting Services for Next.js Apps
Hosting Provider | Key Feature | Recommended For |
---|---|---|
Vercel | Optimized for Next.js | Next.js websites with serverless functions |
Netlify | Easy CI/CD integration | Static sites and serverless functions |
AWS Amplify | Scalable cloud hosting | Websites with heavy traffic and custom backends |