Create React Website with Ai
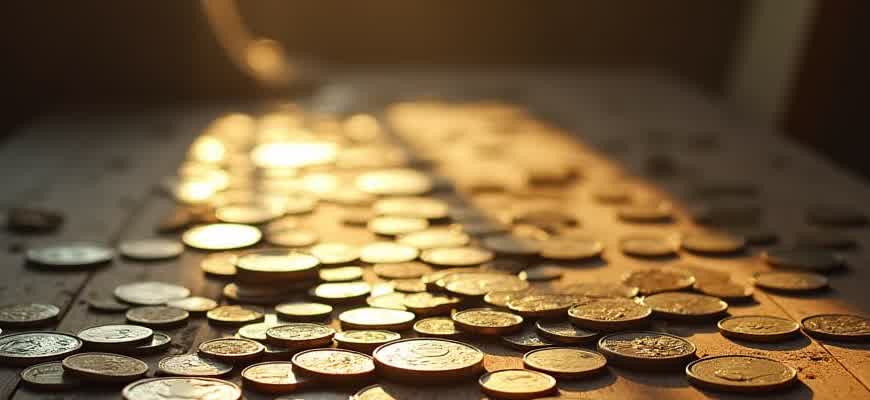
Creating a modern website with React is a powerful way to deliver dynamic user experiences. When combined with AI tools, this development approach can add intelligent features that adapt to user behavior, optimize performance, and automate processes.
To start, you'll need to follow a few essential steps:
- Set up a new React application using create-react-app.
- Integrate AI services through APIs or frameworks like TensorFlow.js or OpenAI.
- Implement AI-powered components for real-time data processing and prediction.
Here's a simple structure of how AI can enhance different aspects of a website:
Feature | AI Integration | Benefit |
---|---|---|
Personalization | AI-powered recommendation system | Tailored content and products for users |
Search Optimization | Natural Language Processing (NLP) | Improved search results accuracy |
Chatbot | AI conversational agents | Instant support and user interaction |
Tip: Leveraging AI libraries with React can significantly reduce development time and provide users with a seamless experience.
Create a React-Based Website Powered by AI
Developing a React website integrated with artificial intelligence involves combining the flexibility of React with the advanced capabilities of AI. This approach allows developers to create dynamic and intelligent web applications that can learn from user behavior, adapt, and provide enhanced user experiences. The integration of AI can range from simple automation to sophisticated machine learning models, all while maintaining React’s component-based structure for efficient development.
To build a React application with AI, one needs to incorporate AI functionalities such as recommendation engines, chatbots, image recognition, or predictive analytics. These features can significantly improve user engagement and provide a personalized experience. Here's how to get started:
Steps to Build a React Website with AI Integration
- Set Up React App: Begin by setting up a React project using Create React App or any other preferred tool.
- Integrate AI Model: Choose an AI tool or framework (like TensorFlow.js, OpenAI, or a custom API) and integrate it with your React app.
- Optimize Performance: Ensure that AI features are optimized for speed and efficiency to avoid slowing down the user experience.
- Deploy & Test: After building the app, deploy it and thoroughly test the AI functionalities with different datasets and scenarios.
To create a truly interactive React website, integrating AI should not only serve functional purposes but also enrich the user's journey.
Examples of AI Features for a React Website
AI Feature | Description | Use Case |
---|---|---|
Chatbots | Automated response systems using natural language processing. | Customer service or support. |
Recommendation Engine | Analyzes user data to suggest personalized content. | E-commerce, media streaming. |
Image Recognition | Identifies objects, faces, or patterns in images using AI algorithms. | Security, social media apps. |
How to Incorporate AI into Your React Website Development
Integrating artificial intelligence into your React application can significantly improve the user experience by enabling features such as personalized recommendations, natural language processing, and smart analytics. AI can help automate repetitive tasks, enhance interactivity, and provide data-driven insights that improve decision-making.
To integrate AI into your React project, you need to choose the right AI tools and libraries. This involves understanding your project's requirements and selecting the appropriate AI models, whether you're working with pre-built APIs or training your own models. Below is a guide on how to proceed.
Steps to Implement AI in React
- Set up an AI model or API: Choose a pre-trained model from services like TensorFlow.js, OpenAI, or IBM Watson. Alternatively, you can train a custom model using frameworks like TensorFlow or PyTorch and deploy it via an API.
- Install AI libraries: Use JavaScript libraries to integrate the model with your React app. Libraries like TensorFlow.js or Brain.js can be installed via npm to work directly in the browser.
- Integrate with React components: Create custom React components that interact with the AI model. For instance, you could use the state to manage the data flow between the model and the component.
Tip: Always test the AI integration thoroughly to ensure that the model is giving accurate results and that the application responds well under different conditions.
AI-Enabled Features in React Websites
- Chatbots: Enhance customer service by integrating AI-powered chatbots that can respond intelligently to user queries.
- Recommendation Systems: Implement recommendation algorithms to suggest products or content based on user behavior and preferences.
- Image Recognition: Use AI to classify and tag images automatically in applications that involve media processing.
- Sentiment Analysis: Analyze user reviews or social media posts to understand customer sentiments and improve your services.
AI Libraries and Frameworks for React
Library/Framework | Use Case |
---|---|
TensorFlow.js | Run machine learning models directly in the browser using JavaScript. |
Brain.js | Simple neural networks for running on the client side. |
OpenAI API | Provide access to powerful language models for tasks like text generation, translation, and more. |
IBM Watson | AI-driven services for natural language processing and data analysis. |
Step-by-Step Guide to Building React Components Powered by AI
Integrating AI into React components can significantly enhance user experience and add dynamic functionality. By leveraging AI-driven APIs or machine learning models, React components can become more intelligent and responsive. This guide will walk you through the process of building React components that utilize AI, focusing on key concepts and implementation steps.
Whether you want to integrate a recommendation engine, natural language processing, or image recognition, AI can add valuable features to your React app. Below is a step-by-step process to help you get started with AI-powered React components.
1. Setting Up the Environment
- Install React - Start by setting up a basic React app using Create React App:
npx create-react-app ai-react-app
- Install Necessary Packages - You will need a package like Axios to make API calls:
npm install axios
- Choose AI Services - Pick a suitable AI service depending on your needs (e.g., Google Cloud AI, OpenAI, etc.). You will need API keys for integration.
2. Building a Simple AI-Powered Component
Now that your environment is ready, let's build a simple AI-powered React component that uses an API to predict text sentiment.
Tip: Ensure you follow API usage guidelines and set up proper authentication (e.g., using environment variables for storing API keys).
- Create a new component in your React app:
src/components/SentimentAnalyzer.js
useState
and useEffect
hooks for state management and data fetching.import React, { useState } from 'react'; import axios from 'axios'; const SentimentAnalyzer = () => { const [text, setText] = useState(''); const [result, setResult] = useState(''); const analyzeSentiment = async () => { const response = await axios.post('AI_API_ENDPOINT', { text }); setResult(response.data.sentiment); }; return (setText(e.target.value)} />); }; export default SentimentAnalyzer;Sentiment: {result}
3. Enhancing the AI Component with Additional Features
Once you have the basic sentiment analysis component, consider adding more AI-powered features to enhance the functionality:
- Real-Time Text Analysis: Use WebSocket for real-time communication between the user and the API.
- Multilingual Support: Integrate a translation service to handle sentiment analysis in multiple languages.
- AI-Generated Suggestions: Based on the sentiment analysis, display recommendations or action items to users.
4. Table of AI Services for React Integration
AI Service | Features | Pricing |
---|---|---|
Google Cloud AI | Sentiment Analysis, Text Analytics, Vision API | Free tier available, pay-as-you-go |
OpenAI | Natural Language Processing, Text Generation | Free tier with limited usage, subscription required for higher usage |
AWS AI | Machine Learning Models, Language Translation, Forecasting | Pay-as-you-go, Free tier for some services |
Conclusion
Building AI-powered React components is an exciting way to enhance your web applications. By following the steps above, you can integrate various AI features into your components and provide a smarter, more engaging user experience. Always ensure you handle data responsibly and choose the right AI services based on your app's requirements.
Leveraging AI Tools for Automated Code Generation in React Projects
As the development of React applications becomes more complex, developers are turning to artificial intelligence to streamline the coding process. AI tools can assist in generating boilerplate code, optimizing logic, and even automating debugging tasks, helping developers save time and focus on higher-level aspects of application design. These tools rely on machine learning models that understand common patterns in React development, making it possible to generate code snippets or entire components based on minimal input.
By integrating AI into a React project, developers can harness the power of automation in various stages of the development cycle. AI tools not only improve the speed of development but also enhance the consistency of code quality. They analyze large datasets of codebases to learn best practices and can suggest improvements or generate code that adheres to coding standards and guidelines.
How AI Tools Automate Code Creation
AI-driven code generation tools offer several benefits, including:
- Automatic generation of repetitive UI components based on user input
- Suggestions for optimizations in existing code
- Real-time error detection and debugging
These tools are particularly helpful when building large-scale React applications that require consistent patterns and best practices across numerous components.
Important: While AI can significantly accelerate the development process, human oversight is still necessary to ensure the final product meets the specific requirements and user needs.
Popular AI Tools for React Development
Several AI-powered platforms are already being used by developers to automate React code generation:
- GitHub Copilot: An AI-powered code completion tool that provides real-time suggestions based on context.
- Tabnine: A code suggestion tool that helps developers by predicting the next line of code or generating entire functions based on the project’s context.
- DeepCode: Uses AI to analyze code for potential bugs and provide recommendations for fixes or improvements.
AI and Code Consistency in React
AI tools also ensure that the generated code maintains consistency across a project. This is achieved through pattern recognition and adherence to best practices, especially in larger codebases where human error might otherwise lead to inconsistencies.
AI Tool | Key Feature | Benefit |
---|---|---|
GitHub Copilot | Real-time code suggestions | Improved coding speed |
Tabnine | Contextual code prediction | Reduced errors and manual input |
DeepCode | Bug detection and recommendations | Enhanced code quality |
Leveraging AI for Predictive Features and Dynamic Content in React Applications
Integrating AI in React websites can significantly enhance the user experience by providing personalized, predictive features and dynamic content. React, with its component-based architecture, allows easy incorporation of AI models, which can adapt content based on user behavior and preferences in real-time. Predictive capabilities can optimize user interactions and content delivery, ensuring that the site remains relevant to the audience's needs.
Artificial intelligence, when combined with React’s reactive UI, can adjust to user inputs, generating dynamic responses that would otherwise require manual updates. By analyzing previous user actions, AI models can predict future behaviors, serving personalized content or offering recommendations, effectively keeping users engaged.
Predictive Features
AI-powered predictive features utilize machine learning algorithms to forecast user actions or preferences. This can result in:
- Dynamic content recommendations based on previous interactions.
- Automatic content updates based on user interest and browsing patterns.
- Real-time updates to enhance user engagement without manual intervention.
For example, e-commerce websites can use predictive algorithms to recommend products based on browsing history, while news sites might tailor article suggestions based on reading behavior.
Dynamic Content Delivery
AI enables websites to deliver dynamic content that adjusts according to the user's needs. This dynamic interaction can include:
- Personalized homepages displaying items or topics of interest.
- Real-time notifications about deals, updates, or new content.
- Auto-generated chatbots that provide real-time support or guidance.
Important: By using AI for dynamic content, websites can maintain high levels of engagement, offering users content that is relevant, timely, and tailored to individual preferences.
Example Implementation
Feature | AI Technology | Benefit |
---|---|---|
Product Recommendations | Collaborative Filtering | Increases sales by recommending products based on user preferences. |
Real-Time Content Updates | Natural Language Processing | Delivers personalized content based on browsing history or preferences. |
Customer Support Chatbots | AI-Powered Chatbots | Improves user experience by providing 24/7 support and guidance. |
Implementing AI-Driven User Interfaces for Enhanced User Experience
Artificial Intelligence (AI) is revolutionizing the way we design user interfaces by making them smarter and more intuitive. AI-driven UI can adapt to user behavior, predict needs, and personalize interactions, which significantly enhances the overall user experience. Through the use of machine learning algorithms and predictive analytics, interfaces become more responsive, anticipating user actions and offering personalized content. These innovations create a seamless, efficient environment for users to interact with applications, increasing satisfaction and engagement.
AI-driven design integrates natural language processing, image recognition, and real-time decision-making to tailor the user journey. By incorporating these technologies, developers can create interfaces that are not only functional but also contextually aware. This approach shifts away from static designs, fostering dynamic experiences that evolve based on user preferences, behavior, and past interactions.
Benefits of AI-Powered User Interfaces
- Personalization: AI can analyze user data to customize experiences, delivering relevant content based on individual preferences and habits.
- Efficiency: AI algorithms automate repetitive tasks, allowing users to focus on more important actions while reducing time spent on routine functions.
- Adaptability: AI-driven systems adjust the interface based on user feedback and interactions, improving user experience over time.
Key Technologies for AI-Driven UI
- Natural Language Processing (NLP): Enables users to interact with interfaces using voice or text commands, enhancing accessibility.
- Machine Learning (ML): Learns from user data to predict actions and improve UI responsiveness.
- Computer Vision: Allows AI to recognize images and visual elements, optimizing the interface based on user context and actions.
AI in Practice: Example Comparison
Traditional UI | AI-Driven UI |
---|---|
User has to manually input information every time. | AI predicts user preferences and autofills data based on past behavior. |
Static interface with limited personalization. | Dynamic interface adapts to user needs, offering personalized content. |
No context-aware adjustments. | Interface adjusts in real-time based on environmental and user data. |
AI-driven interfaces provide not only functionality but also the ability to engage users in a more meaningful way, making technology feel more intuitive and personal.
Optimizing React Websites with AI for Enhanced Performance
When building React websites, optimizing for speed is crucial to improving user experience. Artificial Intelligence (AI) can play a vital role in this optimization process. By automating tasks like image optimization, code splitting, and server-side rendering, AI helps streamline the website's performance, making it faster and more efficient.
AI-driven optimization techniques can analyze real-time data and adapt dynamically, ensuring that the website performs optimally under different circumstances. Here’s a breakdown of how AI can help in improving load times for React applications:
Key AI Optimization Techniques for Faster Load Times
- AI-Based Image Optimization: AI can automatically adjust image resolution based on the user’s device and network conditions, reducing unnecessary file sizes without compromising visual quality.
- Smart Code Splitting: AI can dynamically determine the most appropriate parts of the application to load, splitting large chunks of JavaScript into smaller, more manageable pieces based on user behavior and device characteristics.
- AI-Powered Server-Side Rendering: Server-side rendering (SSR) with AI can prioritize essential content for quick rendering, improving Time-to-First-Byte (TTFB) and reducing perceived loading time.
How AI Enhances React Website Performance
- Predictive Loading: AI analyzes user patterns and predicts the next steps or pages the user will visit, preloading those resources in the background.
- Content Delivery Network (CDN) Optimization: AI helps in selecting the most optimal CDN node based on the user’s location, ensuring faster content delivery.
- AI-Assisted Error Detection: AI tools can automatically detect bottlenecks or performance issues in real-time, providing developers with actionable insights to improve site responsiveness.
AI can optimize load times by making intelligent decisions about which resources to load, when to load them, and how to adjust for network conditions, reducing unnecessary delays.
AI Optimization Strategies at a Glance
AI Optimization Method | Benefit | Impact on Load Time |
---|---|---|
Image Compression | Reduces image size without losing quality | Fastens page load by reducing heavy assets |
Code Splitting | Loads only the required code for a given page | Speeds up initial load by minimizing JavaScript size |
Predictive Resource Preloading | Preloads resources based on user behavior | Decreases perceived load time for the user |
Best Practices for AI-Enhanced Debugging and Testing in React Development
Incorporating AI into the development workflow, especially for debugging and testing, can significantly streamline the process and improve efficiency. For React developers, AI tools offer various ways to automate error detection, enhance unit testing, and ensure that user interfaces behave as expected. However, it is essential to adopt certain practices to fully leverage AI's potential while maintaining high-quality code and minimizing human error.
React applications, with their dynamic and component-based nature, often present unique challenges during the testing and debugging phases. By using AI-assisted tools, developers can detect potential issues like state mismatches, UI inconsistencies, or performance bottlenecks more quickly. Below are some best practices to follow when integrating AI into your testing and debugging routines.
1. Automated Unit Testing with AI
- Utilize AI-powered test generators: AI tools can analyze the codebase and suggest unit test cases based on the logic and components within the React application.
- Prioritize high-risk components: Use AI to identify critical paths or components that may require more testing based on usage frequency or complexity.
- Integrate with CI/CD pipelines: Incorporate AI-driven test suites into continuous integration workflows to ensure tests are run automatically with every code change.
2. Debugging with AI Assistance
- AI-based error tracking: Implement tools like Sentry or Bugsnag, which use AI to automatically categorize and prioritize errors, enabling quicker fixes.
- Leverage machine learning for performance optimization: AI can analyze performance logs and suggest optimizations, such as lazy loading or code splitting, to improve React app efficiency.
- AI-powered code refactoring: Some AI tools can recommend code improvements or even refactor certain sections automatically to enhance readability and performance.
"AI-assisted debugging tools help developers detect subtle issues that may not be visible during manual testing, allowing for faster resolution and more reliable applications."
3. AI-Driven Test Coverage Analysis
Tool | Use Case | AI Feature |
---|---|---|
Testim | Automated UI testing | AI learns from previous test cases to predict future issues and generate new tests. |
Applitools | Visual regression testing | AI analyzes visual differences and identifies potential UI bugs. |