Drag and Drop Website Builder Laravel
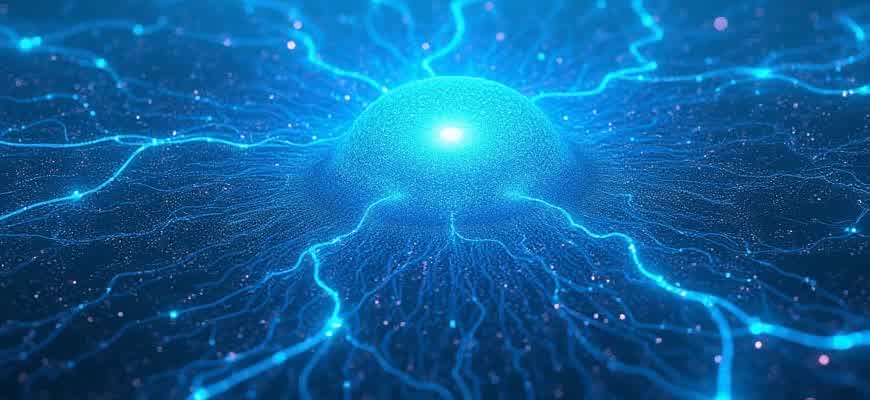
Laravel provides a robust framework for developing dynamic web applications, and when combined with a drag-and-drop functionality, it simplifies user interface creation significantly. By utilizing this feature, developers can create interactive websites that allow users to visually arrange elements without writing complex code. This approach greatly enhances the user experience while offering powerful customization options.
Key Benefits of Integrating Drag-and-Drop with Laravel:
- Enhanced usability for non-technical users.
- Rapid design and prototype building.
- Minimal coding required for interactive layouts.
The core functionality of a drag-and-drop website builder is powered by JavaScript libraries like Vue.js or React, seamlessly integrated into Laravel’s backend. The architecture allows for both flexibility and scalability, making it ideal for developing fully-featured web applications.
"The power of drag-and-drop functionality lies in its simplicity, allowing users to manipulate site content effortlessly without technical knowledge."
Structure of a Drag-and-Drop Builder:
- User authentication and role-based access (Laravel provides built-in authentication support).
- Dynamic content management (such as images, text, and forms).
- Real-time content updates using Laravel’s broadcasting capabilities.
The drag-and-drop builder can be structured using a combination of Laravel's routing and controller functionality alongside front-end tools. It is essential to maintain a clean and organized backend to ensure smooth operation and easy updates.
Comparison of Key Features:
Feature | Traditional Development | Drag-and-Drop Builder |
---|---|---|
Customization | Code-intensive | Minimal code, visual customization |
Development Speed | Slower | Faster due to visual editing |
User Interaction | Requires technical knowledge | Accessible for non-technical users |
Integrating Drag-and-Drop Features into Your Laravel Application
Adding drag-and-drop functionality to a Laravel project can significantly enhance the user experience by making content management more intuitive and interactive. To achieve this, you’ll need to combine frontend JavaScript libraries with Laravel's robust backend features. The integration process involves setting up the necessary JavaScript libraries, defining drag-and-drop events, and ensuring the backend processes data efficiently once the content has been rearranged.
One of the most popular tools for this purpose is the Vue.js framework, often used alongside Laravel for seamless component integration. However, any frontend JavaScript library like React or jQuery UI can be used, depending on your project's needs. Below is a step-by-step approach to adding drag-and-drop functionality to your Laravel project.
Steps to Integrate Drag-and-Drop Functionality
- Install Necessary Packages: Start by installing a drag-and-drop library like Vue Draggable or SortableJS.
- Frontend Integration: Include the drag-and-drop library into your project’s frontend using npm or a CDN. Ensure that the Vue components or JavaScript elements are properly set up to handle the drag-and-drop actions.
- Define Data Models: Create a model in Laravel to manage the content being dragged, for instance, a 'Page' or 'Block' model that stores data to be rearranged.
- Backend Handling: Use Laravel routes and controllers to save the new order of the content after a successful drag-and-drop event. Ensure data is updated in your database based on the new positions.
- Test and Debug: Test the functionality thoroughly on different browsers and devices to ensure smooth interaction and data handling.
Example Implementation
Frontend (Vue Component) | Backend (Laravel Controller) |
---|---|
|
public function updateOrder(Request $request) { foreach ($request->items as $index => $item) { Item::where('id', $item['id'])->update(['order' => $index]); } return response()->json('Order updated'); } |
Ensure that you handle errors properly on both the frontend and backend to prevent data loss or incorrect content ordering.
Customizing the User Interface for Seamless Drag and Drop Interactions
Designing an intuitive and efficient user interface for a drag-and-drop website builder in Laravel requires careful attention to user experience (UX) principles. One of the core aspects is ensuring that the interface allows users to easily manipulate elements without unnecessary complexity. By focusing on clean, responsive design and interactive features, developers can create a smooth and satisfying user experience that encourages engagement and ease of use. A well-optimized interface can dramatically improve workflow, making it easier for users to customize their websites in real time.
Customizing the interface involves creating interactive, draggable components that fit seamlessly into the layout. This can be achieved by utilizing JavaScript libraries like jQuery UI or integrating native Laravel functionality with Vue.js for reactive components. Key elements such as buttons, text fields, and widgets must be designed to move fluidly within the design canvas. The goal is to make the drag-and-drop actions feel natural, responsive, and enjoyable for the end user.
Key Considerations for a Seamless Experience
- Visual Feedback: Provide clear indicators such as hover effects or shadows when elements are dragged. This informs users that an action is possible and that an element is ready to be placed.
- Snapping and Alignment: Ensure that dragged elements automatically align with others in the layout. Snapping functionality can help users position components precisely without requiring manual adjustments.
- Responsiveness: The interface should dynamically adjust to different screen sizes, making it accessible and user-friendly across all devices.
Structure and Functionality
- Use of drag handles: These allow users to initiate dragging actions from any part of a component.
- Placeholder zones: Designate drop zones where elements can be placed, preventing overlap or misplacement of components.
- Contextual options: Enable right-click menus or floating toolbars to provide additional customization options for each component once it has been placed on the page.
To ensure smooth drag-and-drop functionality, test the interface thoroughly across multiple browsers and devices, accounting for various screen sizes and potential performance issues.
Example of Draggable Elements Structure
Element Type | Function | Action |
---|---|---|
Text Block | Display editable text content | Drag to desired location and edit text |
Image Block | Upload and display images | Drag to position, resize, or replace image |
Button | Create interactive buttons | Drag to place, edit label, and link action |
Best Practices for Managing Frontend and Backend Communication in Laravel
When building dynamic web applications with a drag-and-drop website builder using Laravel, ensuring seamless communication between the frontend and backend is crucial. This is especially important when integrating complex features like real-time updates, form submissions, and content management. Laravel, with its robust routing and API features, provides a strong foundation for managing these interactions efficiently. By following best practices, developers can ensure that the system remains scalable and maintainable over time.
Effective communication between the frontend and backend is not only about handling requests but also about maintaining a clean architecture. Laravel offers tools such as AJAX, API routes, and Eloquent ORM to handle data transfer securely and efficiently. Below are several best practices for managing these interactions within a Laravel environment.
1. Utilize API Routes for Clean Separation
One of the most effective ways to manage communication is by using Laravel's API routes. By creating dedicated API endpoints, you can keep the frontend and backend logic separate, allowing each side to focus on its specific concerns.
- Use Resource Controllers: Create resource controllers for managing CRUD operations, making it easier to handle repetitive tasks.
- Define Routes Clearly: Use Laravel's Route::apiResource method to automatically define common routes for RESTful API calls.
- Utilize JSON Responses: Always return data in JSON format for easier consumption by the frontend.
2. Implement AJAX for Real-Time Interactions
AJAX allows you to update parts of a page without refreshing the entire content, offering a smoother user experience. Laravel's support for AJAX via jQuery or vanilla JavaScript makes it easy to implement real-time interactions, such as live form validation, dynamic content loading, and instant feedback on drag-and-drop actions.
- Use Laravel’s CSRF Protection: Ensure that AJAX requests are secured by using Laravel's built-in CSRF protection middleware.
- Handle AJAX Responses Properly: Always send appropriate HTTP status codes and error messages in the response to ensure clear communication with the frontend.
- Optimize Data Handling: Limit the amount of data sent via AJAX to avoid unnecessary load on the server and ensure faster responses.
3. Authentication and Authorization
When dealing with sensitive user data, authentication and authorization are essential. Laravel offers several methods for securing your application, such as token-based authentication or session-based authentication for more traditional web applications.
Important: Always ensure that your API routes are protected with authentication middleware, such as auth:api or auth, to prevent unauthorized access.
Method | Usage |
---|---|
Session-based Authentication | Use when building a traditional web application with Laravel’s built-in session handling. |
API Token Authentication | Use when building single-page applications (SPAs) or mobile apps that require stateless authentication. |
How to Add and Organize Widgets in Laravel's Drag-and-Drop Builder
Integrating widgets into your Laravel-based drag-and-drop website builder can significantly enhance user interaction. With Laravel, the process is streamlined and flexible, enabling developers to manage widgets efficiently. This process involves dragging components into predefined areas on a page and organizing them into a seamless layout. By leveraging the framework's features, developers can implement a highly customizable system that supports a variety of widgets.
To start, adding widgets typically involves selecting a widget from a list and positioning it within a content block. Each widget can have its own configuration options, such as size, content, or style. Once placed, the widgets can be moved or removed based on user preferences. Below is a step-by-step guide on how to add and organize these elements in a Laravel drag-and-drop environment.
Step-by-Step Guide for Adding Widgets
- Choose a widget from the available list in the builder interface.
- Drag the selected widget to the desired section on the page layout.
- Adjust the widget’s properties (such as size, color, or content) through the options panel.
- Save the layout to apply changes to the website structure.
Organizing Widgets
Once widgets are placed, organizing them into logical sections ensures that your site remains functional and visually appealing. Here's how to effectively manage widget placement:
- Group similar widgets into predefined sections or rows for easier management.
- Use nested layouts to create complex designs with minimal effort.
- Drag widgets to reorder them within a container to adjust the flow of content.
- Save layouts after each adjustment to prevent losing changes.
Remember, keeping your widgets organized not only enhances user experience but also improves website performance and scalability.
Table of Widget Types and Functions
Widget Type | Function | Customization Options |
---|---|---|
Text Block | Displays custom text | Font size, color, alignment |
Image Gallery | Shows multiple images in a grid | Grid layout, image size, hover effects |
Form | Captures user input | Field types, validation rules, styles |
Improving Website Performance with Drag and Drop Functionality in Laravel
When developing a drag-and-drop website builder using Laravel, it's crucial to focus on optimizing performance to ensure smooth user interaction and reduce load times. Performance issues often arise when handling large amounts of data or complex interactions, but with the right strategies, these can be minimized effectively. Below are some best practices that help optimize performance when integrating drag-and-drop functionality in Laravel applications.
To enhance the user experience and maintain fast load speeds, developers can focus on both front-end and back-end optimizations. From minimizing unnecessary re-renders to caching dynamic data, there are multiple approaches to increase efficiency and responsiveness in drag-and-drop interfaces.
Backend Optimization Tips
- Efficient Data Handling: Ensure minimal data processing by loading only necessary data on the page. Use Laravel's pagination or lazy loading techniques to avoid fetching excessive data.
- Optimize Database Queries: Use indexing and avoid N+1 query problems. Utilize Eloquent relationships efficiently and consider using raw queries for complex data retrieval.
- Job Queueing: For tasks that can be processed asynchronously (such as updating user settings or saving layouts), implement Laravel’s job queue system to offload work from the main process.
Frontend Optimization Tips
- Limit DOM Manipulation: Avoid excessive DOM updates during drag-and-drop actions. Use libraries like Vue.js or React to handle changes in a more efficient, reactive manner.
- Use Throttling/Debouncing: Apply throttling or debouncing techniques for drag-and-drop events to prevent too many actions from being processed at once.
- Asynchronous Updates: Make sure that drag-and-drop actions, like moving items or resizing elements, are handled asynchronously to keep the UI responsive.
Note: Always test the drag-and-drop functionality with real-world data to identify bottlenecks and optimize areas where performance lags.
Key Performance Metrics
Metric | Best Practice | Tool/Method |
---|---|---|
Load Time | Reduce HTTP requests and optimize asset sizes | Laravel Mix, Webpack |
Database Response Time | Implement caching and optimize queries | Laravel Cache, Eloquent |
Client-side Responsiveness | Minimize DOM manipulations, use efficient rendering | Vue.js, React |
Integrating Live Data Updates with Laravel's Drag-and-Drop Website Builder
In modern web development, providing users with real-time data updates is a key feature for enhancing user experience. When integrating such updates with a drag-and-drop website builder built on Laravel, it becomes crucial to handle both the dynamic nature of the interface and the data flow efficiently. This process involves setting up a system that allows users to modify content visually while ensuring the underlying data is always in sync with the changes made in real-time.
Laravel’s powerful ecosystem offers several tools that can facilitate this integration, such as Laravel Echo for broadcasting events and WebSockets for maintaining live connections. By utilizing these technologies, developers can create an interactive platform where any modification on the frontend, like moving widgets or editing content, triggers immediate updates on the backend without the need for page reloads.
Steps for Integrating Real-Time Data
- Set up Laravel Echo: Install and configure Echo, which will manage the real-time communication between the frontend and the server.
- Create WebSocket Server: Use a WebSocket server to handle live data transmission, ensuring that all data changes are broadcasted to connected clients instantly.
- Use Events and Listeners: Define custom Laravel events that will be triggered whenever a user interacts with the builder, such as dragging a component. Listeners will capture these events and update the database accordingly.
- Frontend Integration: Implement Echo on the client side to listen for broadcasted events and update the user interface dynamically without requiring a page reload.
Real-Time Data in Action
"Integrating real-time updates in a drag-and-drop website builder allows users to see instant changes as they build, resulting in a more interactive and engaging experience."
By combining Laravel's backend capabilities with real-time updates, developers can create websites where content changes immediately reflect on all user interfaces. The following table outlines the basic flow of data interaction:
Action | Frontend Reaction | Backend Response |
---|---|---|
Drag Widget | Widget moves on the screen | Backend updates widget position in database |
Edit Text | Text field changes | Database is updated with new text content |
Save Layout | Visual layout is saved | Database saves the entire layout configuration |
Handling File Uploads and Media Management with Drag and Drop Features
In modern web development, facilitating an intuitive media management system is essential, especially for platforms that involve rich content creation. When implementing drag-and-drop functionality in a Laravel-based website builder, one of the critical aspects to address is the seamless handling of file uploads and media management. This feature allows users to easily upload files, such as images, videos, and documents, directly to the platform with minimal effort. By combining drag-and-drop interaction with server-side handling in Laravel, developers can provide a smooth and efficient user experience.
Managing large volumes of media files is another challenge when creating a website builder. It requires organizing, storing, and retrieving files in a way that supports scalability and user accessibility. Laravel, with its built-in file handling mechanisms, can be integrated with drag-and-drop functionalities, allowing users to manage their assets effectively. Below are several important strategies and features that can be employed to achieve this goal.
Key Strategies for File Uploads and Media Management
- Implementing Dropzone.js: A popular JavaScript library for drag-and-drop file uploads that can be integrated with Laravel to handle media files efficiently.
- Optimizing File Storage: Using Laravel's file storage system (e.g., local, cloud, or S3) ensures that uploaded files are stored securely and can be accessed easily by the application.
- Handling File Validation: Adding validation checks, such as file type and size, ensures only appropriate files are uploaded, preventing potential errors and security risks.
Effective media management requires both frontend and backend integration, ensuring a smooth process for the user while maintaining security and performance on the server side.
Best Practices for Media Management
- Media Library Integration: Use Laravel's built-in media management packages like Spatie Media Library to manage uploaded files, associate them with models, and perform operations like resizing and cropping images.
- Thumbnail Generation: Automatically create thumbnails for images upon upload, which can significantly improve the user interface and experience.
- Database Records for Media Files: Store file paths and metadata in the database for easier file retrieval and management.
File Upload Workflow Example
Step | Action | Laravel Component |
---|---|---|
1 | User drags and drops a file | Dropzone.js + Ajax |
2 | Laravel validates and stores the file | Laravel Validation + File Storage |
3 | File path and metadata saved to database | Laravel Eloquent ORM |