Drag and Drop Website Builder Javascript Library
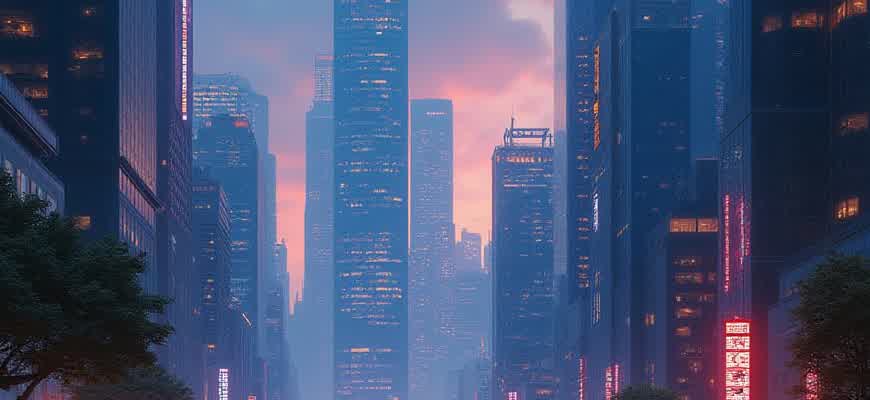
The development of dynamic websites has significantly been simplified with the introduction of interactive tools. A drag-and-drop website builder library in JavaScript offers users a seamless way to construct websites without the need for advanced coding skills. This approach enables both developers and non-developers to design web pages quickly by simply dragging and placing elements onto the canvas. Below is a breakdown of the core features these libraries typically offer:
- Intuitive user interface for easy interaction.
- Pre-designed components such as headers, footers, and buttons.
- Real-time preview of the design.
- Customizable layout options for responsiveness.
Key Benefits:
- Efficiency: Saves time by simplifying the design process.
- Flexibility: Offers customization and easy adjustments.
- User-Friendly: Reduces the need for manual coding.
"This technology revolutionizes the way websites are built, making it possible for anyone to create professional-looking sites without technical expertise."
Many of these libraries also provide a drag-and-drop interface for placing elements directly on the webpage, which can be customized further using JavaScript. Here's a quick look at a simple structure for using such a library:
Element | Action |
---|---|
Text Block | Drag to desired location and edit content |
Image | Drag and set image source |
Button | Place and link to external URL |
How to Integrate a Drag-and-Drop Builder into Your Web Project
To integrate a drag-and-drop builder into your website, you need to start by selecting the appropriate library that aligns with your project requirements. There are various JavaScript libraries available, each with its own set of features, such as user interface customization, event handling, and element management. Some libraries are lightweight and simple, while others offer advanced functionalities like responsive design and real-time updates.
Once you’ve chosen a library, the next step is to embed it into your project. Typically, this involves adding the library's CSS and JavaScript files to your project and initializing the drag-and-drop functionality. The process may vary depending on whether you're using plain JavaScript or a framework like React or Angular. Below are the steps to properly implement a drag-and-drop feature into your web project:
Steps to Integrate
- Install the Library: You can either download the library directly from the official website or use a package manager like npm or yarn. For example, if you're using npm, you would run the following command:
npm install drag-and-drop-library
- Include the Files: Add the necessary CSS and JS files to your project. This could be done via CDN or by linking the local files within your project.
- Initialize the Drag-and-Drop: After including the necessary files, initialize the drag-and-drop feature by targeting the elements you want to be draggable and droppable.
const dragElement = document.querySelector('.drag'); new DragAndDropLibrary(dragElement);
- Customize the Interactions: Depending on the library, you can customize the drag-and-drop behaviors, such as specifying allowed drop zones, controlling the drag sensitivity, or adding visual feedback like dragging animations.
Tip: Make sure to test the integration across multiple browsers and devices to ensure compatibility and a smooth user experience.
Key Considerations
Feature | Considerations |
---|---|
Responsiveness | Ensure the drag-and-drop elements adapt to different screen sizes and orientations. |
Performance | Choose lightweight libraries to ensure optimal loading times and smooth performance. |
Accessibility | Implement keyboard navigation and screen reader support for inclusive user experiences. |
Optimizing Performance of Drag and Drop Features in Web Applications
Efficient performance in drag-and-drop interfaces is crucial for user experience. Web applications that rely on such features must ensure smooth interaction even when handling complex elements or large datasets. There are several strategies to minimize lag and maintain responsiveness while offering users a seamless drag-and-drop experience. These include optimizing rendering, reducing unnecessary event listeners, and minimizing DOM manipulation.
One of the primary considerations is ensuring that the event handling for drag-and-drop operations is optimized. Every drag action triggers a series of events, such as dragstart, dragover, and drop, which can become costly if not handled correctly. These events should be managed with performance in mind, avoiding redundant operations and minimizing computational overhead during interactions.
Techniques to Improve Performance
- Lazy Loading and Virtualization: Only load elements that are visible or near the viewport. This reduces the number of elements in the DOM and helps improve responsiveness.
- Throttling and Debouncing Events: Reducing the frequency of event handling can dramatically improve performance, especially in drag movements where continuous updates are triggered.
- Minimize Layout Reflows: Reflows are expensive operations that occur when an element's size or position is changed. Limit the number of changes that affect layout to reduce reflow costs.
- Use of Document Fragments: When manipulating multiple DOM elements, consider using a document fragment to avoid multiple reflows during batch operations.
Important Considerations
Reducing Event Listeners: Attach event listeners only when necessary. Avoid global event listeners or attach listeners directly to elements that need them. This limits unnecessary event propagation and improves efficiency.
Example of Optimized Drag-and-Drop Structure
Feature | Impact on Performance |
---|---|
Lazy Loading | Significant reduction in initial page load time by only rendering elements in view. |
Throttling Events | Prevents excessive rendering by limiting the number of event triggers per second. |
Minimizing Reflows | Reduces the computational cost by avoiding layout recalculations. |
Customizing UI Elements for Optimal Interaction
Creating an intuitive and visually appealing interface is crucial for any drag-and-drop website builder. The way UI components are presented and interact with users can significantly impact their experience. Customizing elements such as buttons, toolbars, and panels ensures that the user journey remains fluid and enjoyable. In this context, developers must consider the ease of access and clarity of controls for users with varying levels of experience.
Beyond aesthetic customization, functional adjustments to components like widgets, menus, and icons are key to improving usability. A well-designed interface should not only look polished but also provide a seamless workflow, reducing friction and enhancing efficiency for the user.
Key Components to Customize
- Toolbars and Menus – A streamlined toolbar with essential tools can reduce cognitive load, offering quick access to frequently used features.
- Widgets and Panels – Customizable drag-and-drop panels allow users to personalize their workspace, improving interaction and organization.
- Icons and Buttons – Clear, recognizable icons paired with intuitive actions are essential to creating a frictionless user experience.
Best Practices for Customization
- Consistency in Design – Ensure that all UI elements follow a consistent theme in terms of color, shape, and placement for visual harmony.
- Responsiveness – UI components should adapt to different screen sizes, providing a seamless experience across all devices.
- Feedback and States – Clearly indicate active, hover, and disabled states to help users understand their interactions with components.
“The most successful drag-and-drop builders are those that combine simplicity with customization, allowing users to easily tailor their experience while maintaining a user-friendly interface.”
Interactive Component Customization
Component | Customization Option | Impact on User Experience |
---|---|---|
Drag Handles | Adjustable size and positioning | Improves ease of use and precision during interactions |
Tooltips | Customizable content and timing | Enhances clarity and provides helpful guidance without cluttering the interface |
Modals and Popups | Position, transition effects | Improves focus and avoids overwhelming the user with multiple elements at once |
Managing and Storing User-Generated Content with Drag and Drop
Drag and drop functionality allows users to create, edit, and organize content directly on a web interface. As users interact with this type of website builder, their content needs to be efficiently stored and retrieved from a back-end system. Without proper handling, the user experience may suffer, leading to slow performance or data loss. Therefore, managing and storing this content effectively is a key aspect of any drag and drop web builder application.
There are multiple strategies to manage and store user-generated content. Depending on the type of content and the scale of the application, developers can choose between local storage, cloud solutions, or database-driven approaches. Each method offers distinct benefits and limitations that need to be carefully considered.
Best Practices for Storing and Managing Content
- Use Structured Data Storage – Organizing user-generated content in a structured format, such as JSON or XML, simplifies retrieval and management of the content.
- Real-time Synchronization – Implementing real-time data sync ensures that content changes are immediately reflected across all user sessions, minimizing the risk of outdated content.
- Cloud Storage Solutions – Cloud services such as AWS or Google Cloud offer scalability, reliability, and security for user-generated content storage.
Challenges and Solutions
Data Integrity can be a concern when users are allowed to manipulate content in real-time. To ensure content is properly saved and backed up:
- Regularly back up data to avoid loss in case of system crashes.
- Implement version control to track changes made to content, enabling easy rollback if necessary.
"Efficient data storage methods are crucial in maintaining the stability and speed of drag-and-drop builders. Without these, user experience may deteriorate quickly."
Storage Options Comparison
Method | Pros | Cons |
---|---|---|
Local Storage | Fast access, offline availability | Limited storage, security concerns |
Cloud Storage | Scalable, secure, accessible from anywhere | Requires internet connection, potential latency |
Database-Driven | Centralized storage, structured data | Complex to set up, potential scalability issues |
Best Practices for Optimizing Mobile Responsiveness in Drag-and-Drop Builders
Ensuring mobile responsiveness is essential in any web development project, especially when working with drag-and-drop website builders. As the majority of users now access websites through mobile devices, it's crucial to design with mobile-first principles in mind. Here are key strategies to ensure your drag-and-drop builder remains user-friendly and fully functional on mobile screens.
When building responsive websites with a drag-and-drop tool, developers should take into account various factors, such as screen sizes, touch interactions, and performance optimization. Below are some best practices to follow during the development process.
Key Guidelines for Mobile-Friendly Drag-and-Drop Builders
- Responsive Layouts: Use flexible layouts that adjust based on the device's screen width. Ensure grid systems and content blocks scale properly across various screen sizes.
- Touch-Friendly Elements: All interactive elements should be optimized for touch. This includes ensuring buttons, links, and forms are large enough to tap without difficulty.
- Media Queries: Implement media queries to adjust the styles based on the screen size. For example, switching to a single column layout for smaller screens.
- Optimized Images: Use responsive images that load differently based on the screen resolution and size, preventing slower load times on mobile devices.
Considerations for Performance on Mobile
- Lazy Loading: Implement lazy loading for images and other resources. This improves initial page load times, especially on mobile networks.
- Minimize JavaScript: Reduce the use of JavaScript-heavy components or features that can slow down mobile performance. Use lightweight libraries whenever possible.
- Touch Event Handling: Use optimized touch event handling to reduce lag and ensure smooth interactions with the builder's interface.
Tip: Regularly test the website on multiple devices to ensure that the drag-and-drop builder's elements behave as expected across various mobile platforms.
Performance Considerations in Mobile Development
Best Practice | Description |
---|---|
Image Compression | Ensure all images are compressed to reduce loading times without compromising quality. |
Code Minification | Minify CSS, JavaScript, and HTML to reduce file sizes and improve page load speed. |
Offline Support | Implement service workers to provide offline access and enhance the user experience on unstable networks. |
Debugging and Resolving Common Issues in Drag-and-Drop Interfaces
Developing drag-and-drop functionality for web interfaces can often present unique challenges. Understanding the root cause of issues such as unresponsive elements or erratic behavior during dragging is crucial for improving user experience. Below are some of the most common problems developers encounter and how to address them effectively.
While testing drag-and-drop components, it is common to experience misalignments, performance issues, or unexpected behavior when handling DOM elements. These issues can stem from conflicting event handlers, improper positioning, or missing style adjustments. The following troubleshooting strategies will help identify and resolve such problems.
Common Issues and Solutions
- Element Not Moving Smoothly: This typically happens due to incorrect CSS positioning. Ensure that the element being dragged has the correct
position
property set (e.g.,position: absolute
orposition: fixed
). - Drop Zone Not Recognized: If the drop zone doesn’t highlight or accept the dragged element, check that the event listeners for
dragover
anddrop
are correctly implemented and that their default actions are prevented. - Overlapping Drag Events: When multiple draggable elements interfere with each other, use a
z-index
or similar styling techniques to ensure that one element stays on top during interaction.
Steps for Debugging
- Check Event Listeners: Verify that your
dragstart
,dragend
,dragover
, anddrop
event listeners are correctly set up and are not being overwritten or interrupted by other event handlers. - Inspect the Console: Use the browser's developer tools to check for JavaScript errors or warnings that might indicate problems with your drag-and-drop functionality.
- Test with Simplified Layout: Remove extraneous CSS or JavaScript and test the drag-and-drop functionality in isolation to ensure the problem isn't caused by unrelated elements.
Key Considerations for Handling Drag-and-Drop
Issue | Possible Cause | Solution |
---|---|---|
Element Sticking During Drag | Incorrect positioning or missing transform properties | Ensure transform or position properties are properly applied to the dragged element |
Unresponsive Drop Zone | Missing dragover event or preventing default action |
Add event.preventDefault() in dragover to allow dropping |
Unintended Multiple Events | Overlapping or competing event listeners | Use stopPropagation() and ensure event listeners are only attached to the necessary elements |
Tip: Always ensure your drag-and-drop elements have appropriate feedback during interaction. This can improve the user's experience and reduce the chance of encountering bugs related to hidden or unresponsive zones.
Advanced Techniques for Enhancing Your Website Builder
To enhance the functionality of your website builder, incorporating advanced features and customizations is key. By extending the builder’s capabilities, you can create a more flexible, dynamic, and user-friendly platform for your users. This involves integrating additional controls, enhancing UI/UX elements, and allowing for greater customization through custom plugins and APIs.
One way to achieve this is by utilizing event-driven architecture and custom components. By listening for specific actions or events, you can trigger custom behaviors, such as saving data, updating layouts, or integrating third-party services. This method ensures that your builder is responsive and adaptive to user needs.
Techniques for Extending Builder Features
- Custom Component Integration: Add new widgets and modules tailored to your users’ needs, such as custom buttons, media galleries, or dynamic forms. Each component can be configured independently.
- Drag-and-Drop Enhancements: Improve the drag-and-drop functionality by incorporating advanced visual feedback, such as highlighting drop zones or providing custom animations.
- Dynamic Content Handling: Enable real-time content updating through AJAX or WebSockets, allowing content blocks to be modified without page reloads.
Using APIs and External Libraries
Integrating external APIs can provide additional services and extend the builder’s core functionality. For example, you can integrate with APIs for user authentication, cloud storage, or analytics.
- Authentication API: Integrate OAuth or similar services to allow users to securely log in and manage their accounts.
- Cloud Storage API: Connect to cloud services like AWS S3 to store user assets, ensuring seamless file management.
- Analytics API: Incorporate tools like Google Analytics to provide real-time insights into how users interact with the builder.
"Advanced customization capabilities allow developers to turn a simple builder into a highly flexible platform tailored to specific needs, enabling a more engaging experience for end-users."
Example of an Advanced Feature Table
Feature | Description | Integration Method |
---|---|---|
Custom Widgets | Custom components that can be dragged and dropped into the builder | Using React or Vue.js components |
Real-time Updates | Update the website content dynamically without page reloads | AJAX or WebSocket connections |
External API Integration | Link third-party services such as analytics or cloud storage | RESTful API calls |
Security Considerations When Implementing Drag and Drop Features
When integrating drag-and-drop functionality into web applications, it is essential to address security risks to ensure a safe user experience. Implementing this feature improperly may expose vulnerabilities, which could be exploited by malicious actors. It is important to consider both the client-side and server-side security measures to prevent potential attacks such as Cross-Site Scripting (XSS) and unauthorized data manipulation.
To mitigate risks, developers must take into account data validation, content sanitization, and secure handling of user inputs. Proper authentication and authorization mechanisms should be in place to ensure that only authorized users can interact with the drag-and-drop interface, especially when dealing with sensitive data or files. Below are some key considerations when implementing drag-and-drop features securely.
Key Security Measures for Drag-and-Drop Implementation
- Sanitize Inputs: Ensure that any data entered or dragged into the interface is thoroughly sanitized to prevent malicious code from being executed.
- Limit File Uploads: Only allow specific file types to be uploaded, and validate file contents before processing them. This prevents harmful files from being executed on the server or client.
- Cross-Site Scripting (XSS) Protection: Implement measures to prevent script injection attacks by escaping user-generated content and using secure frameworks that mitigate these risks.
Data Validation and Authorization
It is crucial to verify the integrity and authenticity of data during drag-and-drop interactions. Unauthorized users should not be able to drop items or modify data they do not have permission to access. Below are steps for ensuring proper data validation and user authorization:
- Authenticate Users: Implement proper authentication mechanisms (e.g., JWT, OAuth) to ensure that users are identified before interacting with sensitive data.
- Authorization Check: Validate that users have permission to modify or interact with the dropped content. Use role-based access control (RBAC) to manage this.
- Secure Server Communication: Ensure that data is securely transmitted between the client and server by using HTTPS and avoiding the use of unsecured protocols.
Important Notes for Developers
It is crucial to test the drag-and-drop functionality rigorously under various security scenarios to identify any weaknesses in the implementation. Regular security audits should be conducted to keep the application safe from evolving threats.
Security Measure | Description |
---|---|
Sanitize Inputs | Ensure that all user inputs are cleansed to prevent malicious code injection. |
Limit File Uploads | Restrict file uploads to trusted types and validate the content of the files before processing. |
Authorization Checks | Verify that users have the appropriate permissions to interact with dropped content. |