Drag and Drop Website Builder Nextjs
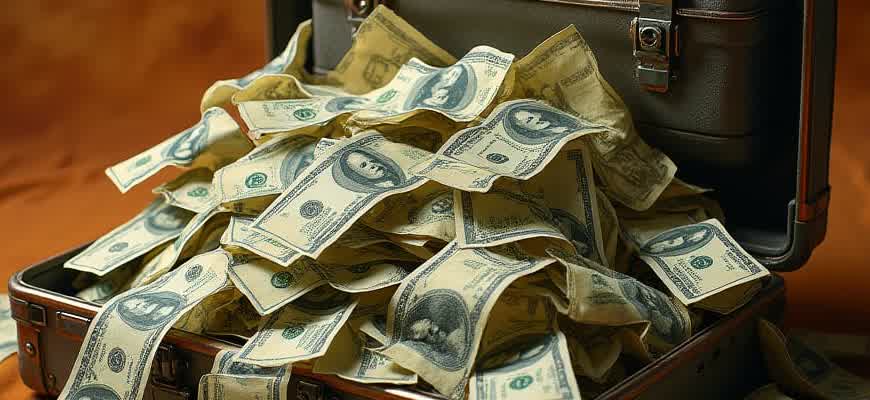
Next.js has become a popular framework for building scalable and performant web applications. One of its strengths is the flexibility it offers for developers to create dynamic and interactive user interfaces. Among the various features that can be incorporated into a Next.js project, a drag-and-drop website builder has gained significant attention due to its ease of use and time-saving advantages.
The integration of a drag-and-drop builder in a Next.js app allows users to visually assemble website components without needing to write code manually. This tool simplifies the development process and can be especially useful for non-developers or teams looking to rapidly prototype websites. Below are some key features that make such a tool indispensable:
- Intuitive interface for easy drag-and-drop interactions
- Real-time previews of changes made during the building process
- Customizable templates and widgets to speed up design
When implementing a drag-and-drop builder in Next.js, several considerations must be made to ensure optimal performance:
"Performance and scalability are key factors when building a website with a drag-and-drop interface, especially when dealing with complex components and a large number of users."
The following table highlights some of the performance considerations for integrating such a builder into a Next.js app:
Factor | Description |
---|---|
Load Time | Efficient asset management and lazy loading for components |
State Management | Optimized handling of component states to avoid unnecessary re-renders |
Server-Side Rendering | Using SSR for faster page loads and better SEO optimization |
How to Integrate Next.js with a Drag-and-Drop Website Builder
Integrating a drag-and-drop website builder into a Next.js application offers an intuitive way for users to create and manage content. This approach leverages the flexibility of Next.js with a visual interface for effortless website design. While Next.js handles server-side rendering and routing, a drag-and-drop builder provides the user-friendly frontend tools to construct layouts dynamically.
To successfully integrate these two technologies, a developer must focus on combining Next.js's efficient rendering capabilities with a user-friendly drag-and-drop interface. The integration typically involves setting up a custom CMS (Content Management System) that communicates with the builder and rendering the designed layouts through Next.js components.
Steps to Implement the Integration
- Set up the Next.js project by creating a new application using `create-next-app`.
- Install and configure the drag-and-drop website builder library, such as React DnD or GrapesJS, within your Next.js app.
- Develop components to handle the dynamic content, ensuring that each component is draggable and editable.
- Implement a CMS or data storage solution to save the user-generated content, which can be fetched and displayed using Next.js's static or server-side rendering.
- Connect the CMS to your builder's output, enabling users to design layouts and store them for future edits.
Note: It’s essential to maintain a responsive and scalable design as the drag-and-drop builder will often generate custom HTML and CSS. Make sure to test the output thoroughly on different devices.
Technical Considerations
Component | Purpose |
---|---|
Next.js | Server-side rendering, routing, SEO optimization |
Drag-and-Drop Builder | UI for visual content creation |
CMS | Stores user-generated content and layouts |
Once the integration is complete, users can seamlessly create and manage websites with drag-and-drop features while Next.js handles the complex backend tasks. By combining these two tools, developers can offer a highly customizable yet user-friendly platform for website creation.
Setting Up a Drag-and-Drop Website Builder on Next.js from Scratch
Building a drag-and-drop website builder with Next.js offers flexibility and scalability, but requires careful consideration of the underlying components. Next.js, with its built-in features such as SSR (Server-Side Rendering) and API routes, is a great choice for building dynamic, fast-loading web applications. To create a drag-and-drop interface, you need to focus on several core parts: user interface components, drag-and-drop functionality, and persistent state management.
The first step in this process is setting up the basic Next.js environment. After installing Next.js and creating your project, the next task is to integrate a library for drag-and-drop capabilities, such as React DnD or React Beautiful DnD. These libraries simplify the drag-and-drop logic, allowing you to focus on the design and functionality of the website builder itself.
Step-by-Step Setup
- Install necessary dependencies:
- Run
npx create-next-app@latest
to create a new Next.js app. - Install React DnD:
npm install react-dnd react-dnd-html5-backend
. - Create the drag-and-drop context:
- Set up a drag-and-drop context to handle state changes when elements are moved.
- Use the
useDrag
anduseDrop
hooks provided by React DnD. - Design the interface:
- Build a simple UI with containers for draggable elements (e.g., text, images, buttons).
- Ensure that each draggable element is correctly styled to provide a visual cue to users.
- Implement state persistence:
- Store the layout and element positions in a state, such as React's
useState
. - Optionally, save the layout to a database or local storage for persistent user data.
Tip: Keep the drag-and-drop interactions as intuitive as possible. A smooth, visual feedback system for dragging and dropping elements will significantly improve the user experience.
Handling State and Persistence
Once the core functionality is in place, managing the state of the builder is crucial. You can use Next.js API routes to store the layout on a server or opt for client-side storage with localStorage for quicker prototyping. As the user arranges elements, updating the state and reflecting those changes in real time should be the main goal.
Storage Method | Pros | Cons |
---|---|---|
localStorage | Fast, easy to implement | Not suitable for large-scale storage |
Server-side (via API routes) | Scalable, persistent across sessions | Requires server setup, slower |
Customizing Components in Next.js with Drag-and-Drop Functionality
Incorporating drag-and-drop functionality into a Next.js project enables users to customize website components effortlessly. This interaction model simplifies the design process, allowing components to be moved, resized, and rearranged visually. By utilizing libraries such as React DnD or React Beautiful DnD, developers can quickly implement dynamic, interactive UIs that offer flexibility to end users without sacrificing performance or scalability.
Next.js, with its server-side rendering and static site generation features, ensures fast load times even with complex interactions like drag-and-drop. The key to effectively customizing components lies in creating reusable building blocks that can be manipulated through simple UI gestures. These components often consist of various interactive elements such as images, text blocks, buttons, and cards, which users can arrange as per their preferences.
Implementing Drag-and-Drop Components
To implement drag-and-drop in Next.js, developers typically follow these steps:
- Install dependencies: Use libraries like React DnD or React Beautiful DnD to handle drag-and-drop functionality.
- Create draggable components: Define which components can be dragged by adding specific properties and event handlers.
- Manage state: Use React’s state management (e.g., useState, useReducer) to track the positions of components during and after the drag action.
- Persist changes: Optionally, save the customized layout in a backend or local storage to maintain the user’s preferences across sessions.
Best Practices for Component Customization
When customizing components in a drag-and-drop interface, it's essential to keep the user experience smooth and intuitive. Here are some best practices:
- Minimize performance overhead: Use lightweight libraries and optimize component rendering to avoid performance bottlenecks during dragging.
- Offer visual feedback: Highlight draggable components with subtle animations or color changes to improve usability.
- Ensure accessibility: Make sure that draggable components are accessible to keyboard users and compatible with screen readers.
Tip: Ensure that your drag-and-drop implementation is mobile-friendly. Use touch events to make components draggable on smaller devices as well.
Example Component Structure
Component | Functionality |
---|---|
Draggable Card | Can be moved within the layout grid and resized by the user. |
Resizable Text Block | Allows users to adjust the size of the text block by dragging its corners. |
Interactive Button | Button actions trigger changes in the layout or content on drop. |
Improving Page Load Efficiency in Drag and Drop Website Builders with Next.js
Optimizing the performance of websites built with drag-and-drop builders on Next.js is crucial for ensuring fast load times. While these tools provide ease of use and flexibility, they can sometimes lead to slower page performance if not properly optimized. By implementing a few strategies, developers can significantly reduce load times while maintaining the rich functionality that users expect from modern web applications.
One of the most effective ways to achieve this is by focusing on the optimization of server-side rendering (SSR) and static site generation (SSG), which are built into Next.js. By using these features efficiently, developers can pre-render pages and serve them as static files, drastically improving page load speed. Additionally, optimizing media assets and deferring non-critical JavaScript can further enhance performance.
Key Optimization Techniques
- Server-Side Rendering (SSR) and Static Site Generation (SSG): Leverage Next.js's built-in SSR and SSG capabilities to ensure that your pages load quickly by pre-rendering content during build time or on the server.
- Lazy Loading of Components: Implement lazy loading for images, components, and other assets to ensure that only visible parts of the page are loaded initially.
- Image Optimization: Use Next.js’s Image component to automatically optimize images, reducing their size and improving load times.
- Minification and Code Splitting: Minify JavaScript and CSS files, and split code into smaller bundles to load only the necessary resources for each page.
Best Practices for Efficient Resource Management
- Use a Content Delivery Network (CDN) to distribute static assets globally, reducing latency.
- Prioritize critical CSS and JavaScript files to load first, deferring non-essential resources.
- Remove unused code and dependencies that may contribute to unnecessary page weight.
“Optimizing your Next.js-based website doesn’t just make it faster; it also improves the overall user experience, leading to better engagement and higher conversion rates.”
Example Table: Impact of Optimization Techniques on Page Load Speed
Optimization Technique | Average Load Time Improvement |
---|---|
Lazy Loading Images | 30% Faster |
SSR and SSG | 50% Faster |
Code Splitting | 20% Faster |
Creating Dynamic Content Blocks with Drag and Drop in Next.js
Building flexible and interactive websites requires tools that enable rapid content management. One effective approach is implementing drag-and-drop functionality for creating dynamic content blocks. In a Next.js application, this can be achieved using a combination of state management and dynamic rendering. With this method, users can intuitively arrange and modify page components without needing to write any code, streamlining the website development process.
By utilizing Next.js's built-in support for server-side rendering (SSR) and React's component-based architecture, you can create highly dynamic, responsive blocks that adapt to various content types. This enables users to design pages on the fly, dragging elements like text boxes, images, and videos into predefined sections of the layout.
Implementation Approach
To start building the drag-and-drop functionality, you can leverage libraries like react-beautiful-dnd or react-dnd. These libraries provide an easy-to-implement solution for handling drag-and-drop interactions within your components.
- Set up drag-and-drop areas where users can place content blocks.
- Define a dynamic state to manage the order and content of blocks on the page.
- Use React components to render each content block based on the current state.
In Next.js, server-side rendering ensures that the content layout is properly indexed by search engines, even when users rearrange elements dynamically. This is crucial for SEO and content discoverability.
Note: Ensure that the drag-and-drop state is synchronized with the backend to persist user changes.
Example Structure
Block Type | Drag Position | Content |
---|---|---|
Text Block | Top Section | Editable content |
Image Block | Middle Section | Dynamic Image |
Video Block | Bottom Section | Embedded Video |
This layout structure allows for easy manipulation of content while maintaining a clean, scalable approach for web development.
Incorporating External Plugins into Your Nextjs Drag-and-Drop Builder
One of the key advantages of using Nextjs for building drag-and-drop website builders is its flexibility to integrate third-party plugins. These plugins can significantly enhance the functionality of your platform, offering features like advanced form handling, dynamic content, or even e-commerce capabilities. By incorporating external libraries or services, you can extend the reach of your builder without reinventing the wheel.
To integrate third-party plugins effectively into a Nextjs project, you need to consider the compatibility of the plugin with server-side rendering (SSR), which is a core feature of Nextjs. Some plugins may not be designed to work in SSR environments, so it's important to check their documentation for specific setup instructions and limitations. This ensures seamless integration and avoids performance issues or crashes.
Steps to Integrate External Plugins
- Identify the plugin or library you want to integrate and ensure it is compatible with Nextjs.
- Install the plugin via npm or yarn, or include it directly in the project if it's a CDN-based solution.
- Use dynamic imports in Nextjs to load plugins only when needed, optimizing the initial load time of your application.
- Test the plugin in both client-side and server-side rendering environments to ensure proper behavior.
Tip: Use dynamic imports to load non-essential plugins only on the client-side to enhance performance.
Example Integration
For instance, to add a rich text editor like Quill.js to your drag-and-drop builder, you can follow these steps:
- Install the Quill.js package using npm or yarn.
- Import the editor into your Nextjs component dynamically using Nextjs's dynamic import feature.
- Include the necessary CSS for Quill.js either globally or scoped within the component.
- Initialize the editor after the component mounts using the useEffect hook to ensure the plugin works properly on the client-side.
Plugin Integration Table
Plugin | Integration Method | Considerations |
---|---|---|
Quill.js | Dynamic import with CSS integration | Client-side only, ensure compatibility with SSR |
Stripe | Install Stripe package and integrate checkout API | Requires backend integration for payment handling |
react-spring | Direct import into components | Performance considerations for large animations |
Securing Your Next.js Website with Drag-and-Drop Features
When developing a website using a drag-and-drop builder with Next.js, it's essential to implement robust security measures to protect both the frontend and backend. While the drag-and-drop functionality simplifies the process of designing and building pages, it also introduces potential vulnerabilities if not handled properly. Proper security ensures that the site remains functional, safe from attacks, and able to handle user data securely.
To secure a Next.js website built with drag-and-drop features, developers must focus on key areas such as authentication, data protection, and API security. This includes securing client-side interactions and ensuring the server-side components remain resilient to various threats like cross-site scripting (XSS) or SQL injection attacks. Let’s dive deeper into the security strategies you can implement.
Key Security Measures for Next.js Websites
- Use HTTPS: Always serve your website over HTTPS to ensure that all data exchanged between the user and your server is encrypted.
- Input Validation: Validate and sanitize all user inputs, especially when using drag-and-drop components that accept user-generated content.
- Authentication & Authorization: Implement proper authentication mechanisms such as OAuth or JWT to control user access and ensure only authorized individuals can modify the website content.
- API Security: Secure your APIs by implementing rate limiting, API keys, and proper validation for all incoming requests.
Handling User Data Safely
Important: Always ensure that user data is stored securely, using encryption both at rest and in transit. Avoid storing sensitive information in places like cookies or local storage without encryption.
- Encrypt sensitive data: Any personal information entered by users should be stored in an encrypted format to prevent unauthorized access.
- Implement CSRF Protection: Cross-Site Request Forgery (CSRF) protection is critical for forms that submit user data. Use tokens to verify the authenticity of requests.
- Access Control: Ensure that users can only access the data they are authorized to view or modify. Implement role-based access controls (RBAC) for better management of permissions.
Additional Tips for Secure Drag-and-Drop Implementations
Security Aspect | Best Practice |
---|---|
Input Validation | Sanitize user-generated content to prevent XSS attacks. |
Session Management | Use secure, HttpOnly cookies for session tokens to prevent hijacking. |
Third-Party Libraries | Ensure all external libraries used for drag-and-drop functionality are up to date and well-maintained. |
Scaling a Next.js Drag-and-Drop Website for High Traffic
As your drag-and-drop website built with Next.js begins to attract more users, ensuring it can handle a surge in traffic becomes a top priority. High traffic websites demand optimized performance to maintain speed, stability, and responsiveness. Next.js offers various built-in tools and features that can significantly help in scaling your site effectively.
Understanding the key factors affecting scalability and implementing strategies to address them can prevent slow load times and downtime. Let’s explore the essential techniques and approaches to handle high traffic for your Next.js-based drag-and-drop website.
Key Strategies for Scaling
- Server-Side Rendering (SSR) and Static Site Generation (SSG): Utilizing Next.js' SSR and SSG capabilities ensures that content is served efficiently and quickly, especially for high-traffic pages.
- Optimizing API Routes: Keep your API routes optimized for minimal database queries and use caching mechanisms to avoid excessive load during traffic spikes.
- Leveraging CDNs: Use Content Delivery Networks to offload static assets and media, ensuring faster delivery and reduced server load.
- Edge Functions: Deploy edge functions to reduce latency by executing code closer to the user’s geographical location.
Performance Optimization Tips
Important: Always prioritize performance for high-traffic scenarios by minimizing JavaScript bundles and optimizing images.
- Implement image optimization using Next.js’ built-in Image component to serve responsive, compressed images.
- Enable lazy loading for offscreen images and components to reduce initial page load time.
- Optimize third-party scripts and defer their execution until necessary to avoid blocking rendering.
Database and Caching Solutions
Method | Description | Benefits |
---|---|---|
Database Sharding | Splitting the database into smaller, more manageable pieces for efficient data retrieval. | Improved performance by reducing the load on any single database server. |
Redis Caching | Using an in-memory data structure store for fast data retrieval. | Reduced database calls and faster response times during high traffic periods. |
Edge Caching | Cache content at edge locations to reduce server load. | Improved scalability and faster content delivery. |