Drag and Drop Website Builder Python
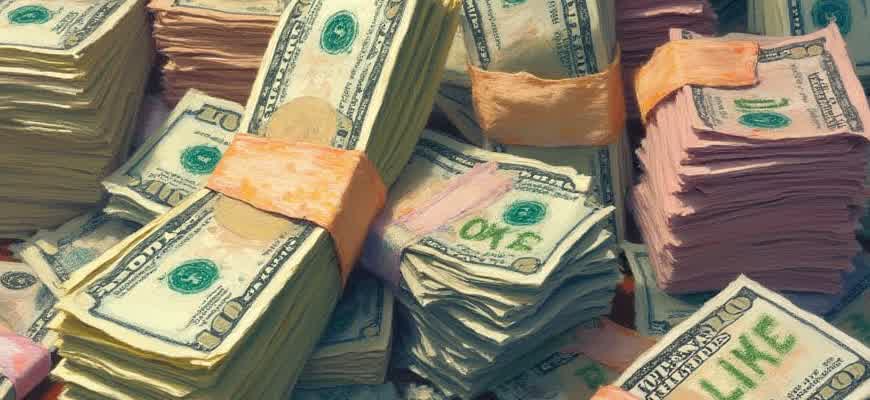
Creating a web-based tool that allows users to design websites using drag-and-drop functionality can be an excellent solution for non-technical users. Python, with its versatility and rich ecosystem of libraries, offers an ideal foundation for building such platforms. By leveraging frameworks like Flask or Django and front-end libraries like React or Vue.js, developers can create an interactive user interface that simplifies website creation.
Key components for a drag-and-drop website builder:
- Back-end framework (Flask, Django) for managing server-side logic.
- Frontend library (React, Vue.js) to handle user interactions in the browser.
- File management system to handle asset uploads and saving user data.
- Real-time preview system to instantly display changes made by the user.
A drag-and-drop builder improves user experience by allowing instant modifications without writing code.
The core of such a tool lies in the ability to handle the user’s visual interaction. The drag-and-drop functionality can be achieved using JavaScript or Python's web libraries that facilitate DOM manipulation. Moreover, integrating a grid or layout system, such as CSS Grid or Flexbox, enhances the flexibility and responsiveness of the web pages being built.
Essential features to implement:
- Element library with pre-designed templates for easy drag-and-drop.
- Customizable styles and content management for each element.
- Publish feature to deploy the site to a live server.
Feature | Description |
---|---|
Drag-and-Drop Interface | Allows users to easily move elements on the canvas. |
Real-Time Preview | Shows changes instantly to the user without needing to refresh the page. |
Setting Up a Drag and Drop Interface in Python
Creating a drag-and-drop interface in Python typically involves leveraging GUI libraries such as Tkinter, PyQt, or Kivy. These tools allow developers to build interactive and user-friendly applications without having to write complex event-handling logic manually. To implement a drag-and-drop system, it is essential to handle mouse events effectively and manage widget placements dynamically.
One of the primary steps in setting up this feature is selecting the right library. Tkinter is often chosen for its simplicity, while PyQt and Kivy provide more advanced features. This process usually begins by setting up a basic window where users can interact with draggable elements, followed by configuring the drag and drop behavior itself.
1. Setting Up a Basic Window
- Start by initializing the main window using the chosen GUI framework.
- Create draggable widgets (e.g., buttons, images) that will be moved within the interface.
- Set up mouse event listeners to capture drag actions.
2. Implementing Drag and Drop Functionality
- Define the actions that will trigger a drag event, such as a mouse press on a draggable element.
- Track the mouse movements while the element is being dragged and update the widget’s position accordingly.
- Drop the element at the desired location by detecting when the mouse button is released.
3. Example with Tkinter
In Tkinter, drag-and-drop functionality can be achieved by binding mouse events to widgets. The example code for moving a button would look like this:
Code |
---|
import tkinter as tk def on_drag(event): widget.place(x=event.x_root, y=event.y_root) root = tk.Tk() widget = tk.Button(root, text="Drag me") widget.place(x=50, y=50) widget.bind(" |
This example demonstrates a button being dragged within the window using mouse motion.
Integrating Backend Logic with Frontend Drag-and-Drop Elements
Integrating backend functionality with frontend drag-and-drop components is essential for creating interactive, dynamic web applications. This integration ensures that user actions on the interface trigger the correct backend processes and provide real-time data manipulation, all while maintaining an intuitive user experience. To achieve this, developers often rely on technologies like WebSockets or REST APIs to bridge the communication between the client-side UI and the server-side logic. This allows for seamless updates and ensures that the user's actions on the frontend reflect immediately on the backend, creating a responsive environment for managing content or user interactions.
The primary challenge in this integration lies in ensuring the backend logic is flexible enough to handle varying frontend interactions while maintaining system performance and security. Data from drag-and-drop actions, such as the positioning of elements or the order of items, needs to be accurately captured and sent to the server. Additionally, server responses must be handled efficiently to avoid UI lags or delays. This requires effective handling of asynchronous requests and careful synchronization between frontend events and backend processes.
Steps for Efficient Backend-Frontend Integration
- Event Handling: On the frontend, JavaScript is commonly used to capture drag-and-drop events and initiate API calls to send relevant data to the backend. Each action, such as dragging a component, must trigger an event handler that packages the data and sends it via HTTP requests.
- API Communication: RESTful APIs or GraphQL endpoints are used to interact with the backend. These APIs should be designed to handle complex operations like updating databases or returning dynamic data based on the user's interaction with the interface.
- Data Persistence: The backend must ensure data integrity and persistence. When drag-and-drop events update the layout or structure of content, the backend must save these changes and respond with the updated data.
Efficient backend integration requires maintaining a balance between real-time responsiveness on the frontend and robust data management on the backend. Asynchronous processing plays a key role in ensuring that the UI remains fluid while backend processes are carried out.
Technologies for Seamless Integration
- JavaScript & Frameworks: Frontend JavaScript libraries like React, Vue, or Angular can be used to implement drag-and-drop features. These libraries allow for easy state management and handling dynamic UI updates.
- Node.js & Express: On the server side, Node.js and Express are commonly used to create fast, asynchronous APIs capable of handling real-time data from drag-and-drop operations.
- WebSockets: For real-time interaction, WebSockets provide full-duplex communication between the client and server, making it ideal for applications that require continuous updates.
Example Backend-Frontend Data Flow
Frontend Action | Backend Response |
---|---|
User drags and drops a content block | Backend receives the new block position and updates the database |
Content block is removed | Backend deletes the block data from the server |
User edits content within a block | Backend processes the updated content and returns the confirmation |
Optimizing Drag-and-Drop Performance for High-Traffic Websites
For websites with high traffic, ensuring that the drag-and-drop functionality remains responsive and smooth is essential for providing a positive user experience. As the complexity of elements on the page increases, so does the potential for performance bottlenecks. It's crucial to implement optimization strategies that keep page load times fast and interactions fluid, even under heavy traffic.
Optimizing the drag-and-drop experience requires focusing on both front-end and back-end performance. This includes minimizing unnecessary DOM manipulation, optimizing event listeners, and leveraging caching techniques to reduce server load. Here are key strategies to enhance performance:
Optimization Strategies
- Lazy Loading Elements: Load only the necessary elements when required, especially for pages with a large number of draggable objects.
- Virtualization of DOM Elements: Instead of rendering every element, dynamically render only those currently visible in the viewport.
- Minimize Reflows and Repaints: Batch DOM updates to avoid multiple reflows during drag operations, which can significantly slow down performance.
- Efficient Event Handling: Use passive event listeners where possible to improve responsiveness and prevent unnecessary event throttling.
Server-Side Optimization
On the server side, caching plays a critical role in reducing server load during high-traffic events. By storing frequently used data or elements locally, websites can minimize the number of requests to the backend, improving both speed and user satisfaction.
"By offloading certain operations to the client-side, such as processing drag events and storing temporary states, you reduce the server burden and improve overall performance."
Performance Monitoring and Tools
Regularly monitoring performance using tools like Chrome DevTools or Lighthouse is vital. These tools allow you to identify performance bottlenecks, such as excessive memory usage or slow JavaScript execution, which can degrade drag-and-drop responsiveness.
Tool | Usage |
---|---|
Chrome DevTools | Inspect and debug performance, memory usage, and DOM rendering issues in real-time. |
Lighthouse | Audit overall website performance and identify areas for improvement. |
Creating Customizable Templates for User Websites
When building a drag-and-drop website builder, offering customizable templates is crucial for users who want to create unique websites. These templates should provide the foundational layout and structure, while allowing users to modify various elements to meet their needs. A flexible design framework will make it easier for users with little to no coding experience to create professional-looking websites without starting from scratch.
Customizable templates should be designed with a range of pre-built sections that can be easily adjusted. The templates should allow for text, image, and layout alterations, enabling users to quickly change colors, fonts, and placements. Offering different categories such as business, portfolio, and personal templates ensures that every user finds a fitting starting point for their project.
Key Features of Customizable Templates
- Predefined grid systems for easy layout control
- Ability to adjust headers, footers, and navigation bars
- Flexible content blocks that can be rearranged or deleted
- Style customization for colors, fonts, and spacing
Incorporating flexible content management tools can further enhance template customization. Users should be able to drag and drop elements to change their placement, or add and remove sections depending on the type of website they wish to build.
By offering a wide variety of templates, website builders can cater to diverse user needs, ensuring that everyone–from hobbyists to professionals–can create a website that suits their vision.
Example of a Template Structure
Section | Customizable Features |
---|---|
Header | Logo, Navigation Menu, Background Color |
Footer | Text, Social Media Links, Copyright Information |
Content | Text, Images, Video, Background Color |
Sidebar | Widgets, Links, Call-to-Action Buttons |
Providing these options allows users to easily personalize their sites without feeling restricted by rigid templates. This flexibility is key to enhancing the user experience and ensuring that the website builder meets diverse needs.
Managing User Inputs and Processing Form Data with Python
When building a drag-and-drop website builder, handling user input and form submissions is a crucial aspect of providing dynamic functionality. Python, with frameworks like Flask or Django, offers powerful tools to efficiently manage these processes. Proper validation and storage of user data ensure a seamless user experience and maintain application integrity.
Form submissions are typically processed using POST requests, and Python frameworks allow easy parsing of this data. In addition, security measures like sanitizing input and validating data types help prevent malicious attacks and erroneous data entry.
Data Handling Process
- Input Collection: Users submit forms with their data (e.g., text fields, checkboxes, radio buttons, etc.). The server receives this input as part of an HTTP POST request.
- Validation: Upon receiving the data, it is important to validate the inputs. This step ensures that the information is in the correct format and meets predefined criteria (e.g., email format, password strength).
- Processing: After validation, the server processes the data, which may include saving it to a database, sending a confirmation email, or performing other actions.
- Response: Once processed, a response is sent back to the user, confirming successful submission or indicating any errors.
Best Practices for Secure Form Handling
- Sanitization: Always sanitize user inputs to avoid SQL injections and other security vulnerabilities.
- CSRF Protection: Implement CSRF tokens to prevent cross-site request forgery attacks on forms.
- Secure Storage: Store sensitive information like passwords using encryption techniques such as bcrypt or PBKDF2.
It is essential to follow these best practices to ensure the reliability and security of user data in web applications.
Example: Storing User Data in a Database
Form Field | Data Type | Validation Rule |
---|---|---|
Name | String | Non-empty, alphabets only |
String | Valid email format | |
Password | String | Minimum 8 characters, includes letters and numbers |
Best Practices for Ensuring Cross-Browser Compatibility in Drag-and-Drop Builders
When developing a drag-and-drop website builder, ensuring that the platform works seamlessly across different web browsers is crucial. Browsers interpret and render code differently, so it’s essential to consider how your builder’s interface and functionality behave in various environments. A consistent user experience is key, as customers will use the tool across different devices and browser versions. To mitigate compatibility issues, developers must follow specific practices during the creation and testing phases.
One of the primary concerns when building drag-and-drop tools is JavaScript performance across browsers. While modern browsers generally support JavaScript well, older versions or less common browsers may struggle with some functionalities. Therefore, compatibility testing should cover a wide range of browsers, from the latest versions of Chrome and Firefox to older ones like Internet Explorer, to ensure that no user is left behind.
Key Techniques for Cross-Browser Support
- CSS Prefixes: Use vendor-specific prefixes to ensure that CSS properties render properly in different browsers.
- Progressive Enhancement: Start with a baseline that works universally, then add more advanced features for browsers that support them.
- Feature Detection: Use tools like Modernizr to check if a browser supports a specific feature before trying to implement it.
- Polyfills: Include polyfills for unsupported features to ensure that older browsers can still function correctly.
Testing Strategies
- Automated Testing: Leverage browser automation tools such as Selenium to test various interactions and ensure compatibility across browsers.
- Manual Testing: Test the drag-and-drop functionality on real devices and browsers to check for inconsistencies in behavior.
- Cross-Browser Tools: Use services like BrowserStack to run your application in different browser environments without needing physical access to each one.
Tools and Frameworks to Facilitate Cross-Browser Development
Tool | Description |
---|---|
Autoprefixer | A CSS post-processor that automatically adds the necessary vendor prefixes to CSS rules. |
Modernizr | A JavaScript library that helps detect features available in the user's browser, enabling conditional loading of resources. |
Can I Use | An online tool that provides compatibility data for HTML, CSS, and JavaScript features across different browsers. |
Important: Regular testing and adherence to web standards can greatly reduce browser-specific bugs and ensure a smooth user experience across all platforms.
Testing and Debugging Drag-and-Drop Features in Python
Ensuring the smooth functionality of drag-and-drop features in a website builder built with Python involves rigorous testing and debugging. The drag-and-drop interaction should feel intuitive and seamless, and any malfunctions can significantly affect the user experience. To achieve this, developers must implement thorough testing processes that simulate real-world use cases and debug any issues that arise during development. Python's testing frameworks such as pytest and unittest are useful in this context for automating tests and ensuring that all components work as expected.
During testing, it is important to check various aspects of the drag-and-drop interaction, such as responsiveness, element positioning, and handling of user input. This includes verifying that elements are properly dragged, dropped, and aligned. Debugging this functionality often requires a combination of traditional logging and utilizing Python-specific tools such as pdb for step-by-step code inspection. Moreover, edge cases such as dragging over non-droppable areas or rapidly dragging multiple elements need special attention.
Key Testing Considerations
- Element Dragging: Ensure that elements can be grabbed and moved smoothly without any flickering or stuttering.
- Element Placement: After dropping, verify the dropped element’s position is accurate according to the layout.
- Edge Cases: Test for rapid dragging, unexpected input, and dragging outside the allowed area.
- Compatibility: Ensure that the drag-and-drop functionality works across different devices and browsers.
Debugging Process
- Enable logging to track event triggers during the drag-and-drop process.
- Utilize Python's pdb to step through the code and identify where the process fails.
- Test component interaction by isolating drag-and-drop logic from other unrelated features.
- Use unit tests to isolate and test individual functions of the drag-and-drop system.
Important: Always test for responsiveness, especially when working with dynamic layout changes or resizing elements during drag-and-drop operations.
Common Debugging Pitfalls
Issue | Possible Cause | Solution |
---|---|---|
Element not moving smoothly | Improper mouse event handling | Check for missing event listeners or delays in event updates |
Dropped element not aligned | Incorrect coordinates during the drop | Check element position calculations and drop target calculations |
Elements not interacting | Wrong DOM updates | Ensure that the drag-and-drop logic correctly manipulates the DOM after each interaction |
Monetization Strategies for a Python-Based Drag and Drop Website Builder
When developing a drag-and-drop website builder using Python, monetizing the platform can be approached in various ways. These methods allow developers to leverage their tools for financial gain while offering value to users. By considering multiple revenue models, you can cater to different market needs and user preferences.
Implementing a combination of monetization strategies ensures both a stable income stream and flexibility for users. Below are some potential approaches to consider when monetizing a Python-powered website builder.
Revenue Models for Python Website Builders
- Freemium Model: Provide a free version with limited features and offer a premium version with additional functionalities.
- Subscription Fees: Charge users on a monthly or annual basis for access to the platform, providing different tiers based on features.
- Template and Plugin Sales: Offer users a marketplace for buying custom templates, plugins, and widgets.
- Advertising: Display third-party ads on the platform, generating revenue from ad impressions or clicks.
Important Considerations
To successfully monetize, it is essential to strike a balance between offering valuable free services and compelling paid options. Focusing on user experience and seamless integration with payment systems can greatly impact the success of your model.
Pricing Strategies and User Tiers
Plan | Features | Price |
---|---|---|
Free | Basic features, limited templates | $0 |
Pro | Advanced features, unlimited templates, premium support | $15/month |
Enterprise | Custom features, dedicated support, API access | Custom Pricing |